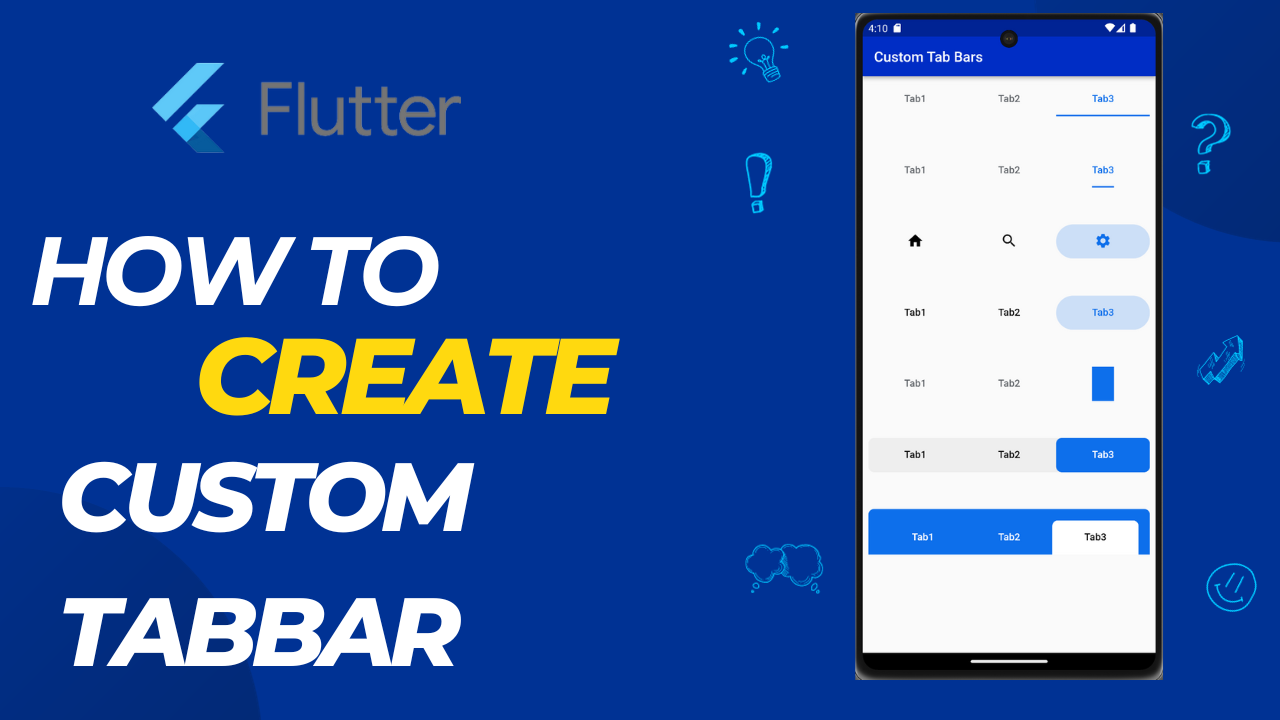
Create Custom Flutter Tabbar:In this section, we are going to learn how the tab bar works in Flutter. The tabs are mainly used for mobile navigation. The styling of tabs is different for different operating systems. For example, it is placed at the top of the screen in android devices while it is placed at the bottom in iOS devices.
Working with tabs is a common pattern in Android and iOS apps that follow the Material Design guidelines. Flutter provides a convenient way to create a tab layout. To add tabs to the app, we need to create a TabBar and TabBarView and attach them with the TabController. The controller will sync both so that we can have the behavior which we need.
Step 1: First, you need to create a Flutter project in your IDE. Here, I am going to use Visual Studio.
Step 2: Open the app in Visual Studio and navigate to the lib folder. Inside the lib folder, create two dart files and named it FirstScreen and SecondScreen.
Write the following code in the FirstScreen:
import 'package:demoproject/tabs.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
fontFamily: 'Poppins',
primarySwatch: Colors.blue,
),
home: TabPage(),
);
}
}
This sample showcases nested Material 3 TabBars. It consists of a primary TabBar with nested a secondary TabBar. The primary TabBar uses a DefaultTabController while the secondary TabBar uses a TabController.
- TabBar.secondary, for a secondary tab bar.
- TabBarView, which displays page views that correspond to each tab.
- TabController, which coordinates tab selection between a TabBar and a TabBarView.
Step 1:
Now to build the TabBar layout in your application the first thing you will need is the Controller. There are two ways to do it either you can use the DefaultTabController or you can build your own TabController.
Step 2:
The next step is the TabBar itself which I discuss earlier, customize your TabBar the way that best suits you according to the parameters mention above in the constructor. In the above TabBar constructor, I mention what value each takes in or what widget needs to be used along with that property. Additionally, the onTap() function can use if you want to alert users by showing a dialogBox or a snackBar or route to another page.
Step 3:
As a required property of TabBar is a list of Tabs, it basically means how many pages are you create accordingly you need to add the Tabs and customize the Icon and Label of the Tabs with the constructor given below and refer to the image of TabBar layout overview.
Full Code:Create Custom Flutter Tabbar
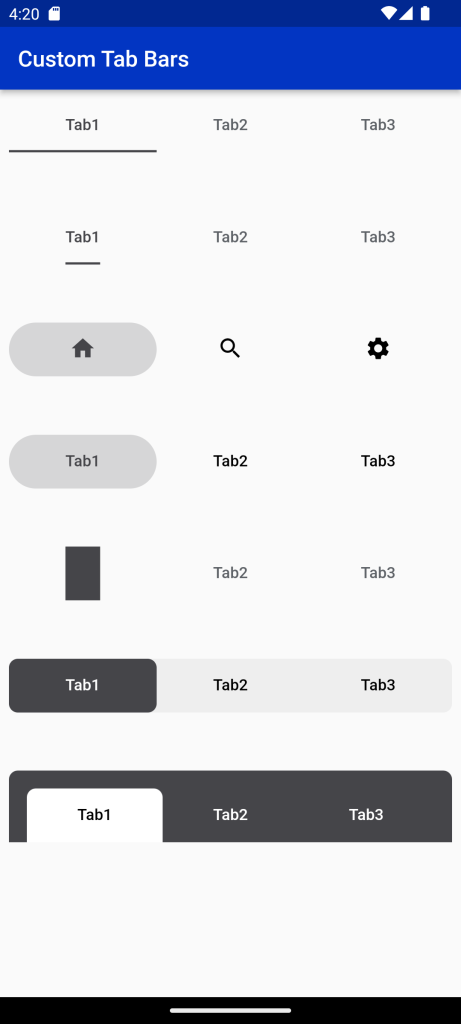
import 'package:demoproject/Constant/color.dart';
import 'package:flutter/material.dart';
class TabPage extends StatefulWidget {
const TabPage({Key? key}) : super(key: key);
@override
_TabPageState createState() => _TabPageState();
}
class _TabPageState extends State<TabPage> with SingleTickerProviderStateMixin {
late TabController _tabController;
final _selectedColor = Color(0xff1a73e8);
final _unselectedColor = Color(0xff5f6368);
final _tabs = [
Tab(text: 'Tab1'),
Tab(text: 'Tab2'),
Tab(text: 'Tab3'),
];
final _iconTabs = [
Tab(icon: Icon(Icons.home)),
Tab(icon: Icon(Icons.search)),
Tab(icon: Icon(Icons.settings)),
];
@override
void initState() {
_tabController = TabController(length: 3, vsync: this);
super.initState();
}
@override
void dispose() {
super.dispose();
_tabController.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Blue,
title: Text("Custom Tab Bars"),
),
body: Padding(
padding: const EdgeInsets.all(8.0),
child: ListView(
children: [
///Default Tabbar with full width tabbar indicator
TabBar(
controller: _tabController,
tabs: _tabs,
labelColor: _selectedColor,
indicatorColor: _selectedColor,
unselectedLabelColor: _unselectedColor,
),
SizedBox(
height: 20,
),
///Default Tabbar with indicator width of the label
TabBar(
controller: _tabController,
tabs: _tabs,
labelColor: _selectedColor,
indicatorColor: _selectedColor,
unselectedLabelColor: _unselectedColor,
indicatorSize: TabBarIndicatorSize.label,
),
SizedBox(
height: 20,
),
/// Custom Material Design tabbar used in google's products
TabBar(
controller: _tabController,
labelColor: _selectedColor,
unselectedLabelColor: _unselectedColor,
indicatorSize: TabBarIndicatorSize.label,
indicator: BoxDecoration(
color: _selectedColor,
),
tabs: _tabs,
),
SizedBox(
height: 20,
),
/// Custom Tabbar with solid selected bg and transparent tabbar bg
Container(
height: kToolbarHeight - 8.0,
decoration: BoxDecoration(
color: Colors.grey.shade200,
borderRadius: BorderRadius.circular(8.0),
),
child: TabBar(
controller: _tabController,
indicator: BoxDecoration(
borderRadius: BorderRadius.circular(8.0),
color: _selectedColor),
labelColor: Colors.white,
unselectedLabelColor: Colors.black,
tabs: _tabs,
),
),
SizedBox(
height: 20,
),
/// Custom Tabbar with solid selected bg and transparent tabbar bg
Container(
height: kToolbarHeight + 8.0,
padding:
const EdgeInsets.only(top: 16.0, right: 16.0, left: 16.0),
decoration: BoxDecoration(
color: _selectedColor,
borderRadius: BorderRadius.only(
topLeft: Radius.circular(8.0),
topRight: Radius.circular(8.0)),
),
child: TabBar(
controller: _tabController,
indicator: BoxDecoration(
borderRadius: BorderRadius.only(
topLeft: Radius.circular(8.0),
topRight: Radius.circular(8.0)),
color: Colors.white),
labelColor: Colors.black,
unselectedLabelColor: Colors.white,
tabs: _tabs,
),
),
SizedBox(
height: 20,
),
/// Custom Tabbar with transparent selected bg and solid selected text
TabBar(
controller: _tabController,
tabs: _iconTabs,
unselectedLabelColor: Colors.black,
labelColor: _selectedColor,
indicator: BoxDecoration(
borderRadius: BorderRadius.circular(80.0),
color: _selectedColor.withOpacity(0.2),
),
),
SizedBox(
height: 20,
),
TabBar(
controller: _tabController,
tabs: _tabs,
unselectedLabelColor: Colors.black,
labelColor: _selectedColor,
indicator: BoxDecoration(
borderRadius: BorderRadius.circular(80.0),
color: _selectedColor.withOpacity(0.2),
),
),
]
.map((item) => Column(
/// Added a divider after each item to let the tabbars have room to breathe
children: [
item,
Divider(
color: Colors.transparent,
)
],
))
.toList(),
),
),
);
}
}
For More: To know about showModalBottomSheet
Leave a Reply