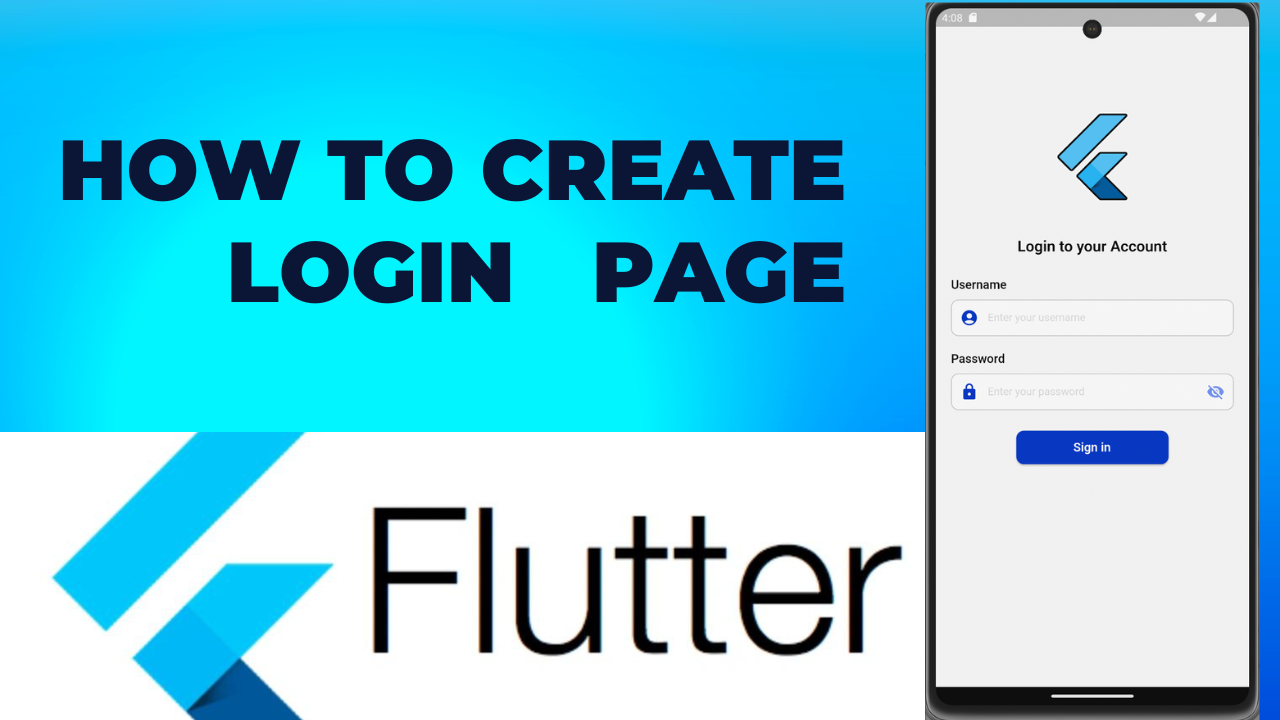
Login Page in Flutter:To prevent unauthorized access to personal information, it is a good practice to authenticate users before granting access to websites, mobile applications, and computer applications, after then register and then login. In this article, we are going to explain how to build a Design Login page user interface. We have used the TextField widget, for user input as Username/Email, and Password. And below of password TextField to create the option Forget Password. Button widget, to show action.
Create new Project
Open the main.dart file locates in the lib folder in a text editor or Visual Studio Code. Change the application logic to increase the counter by 2 instead of 1 if you click the button.
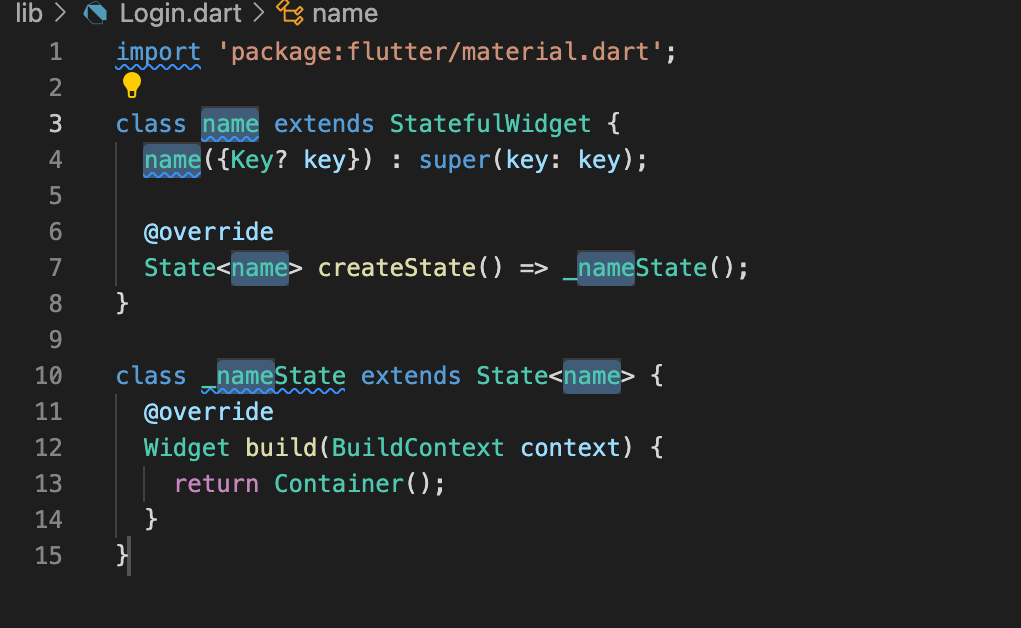
Create new file in lib folder by saving the file name as Login.dart
Open Login.dart file type the following.
- Create a new subclass of StatefulWidget and its associated State subclass.
Type stf or stl in the ide to auto-import all the code necessary to write a stateful or stateless widget. When the shortcut is select, all the code appears with 5 cursors to name your new widget as you need.
class name extends StatefulWidget {
name({Key? key}) : super(key: key);
@override
State<name> createState() => _nameState();
}
class _nameState extends State<name> {
@override
Widget build(BuildContext context) {
return Container();
}
}
In that inside the container we use to start coding for the login page.
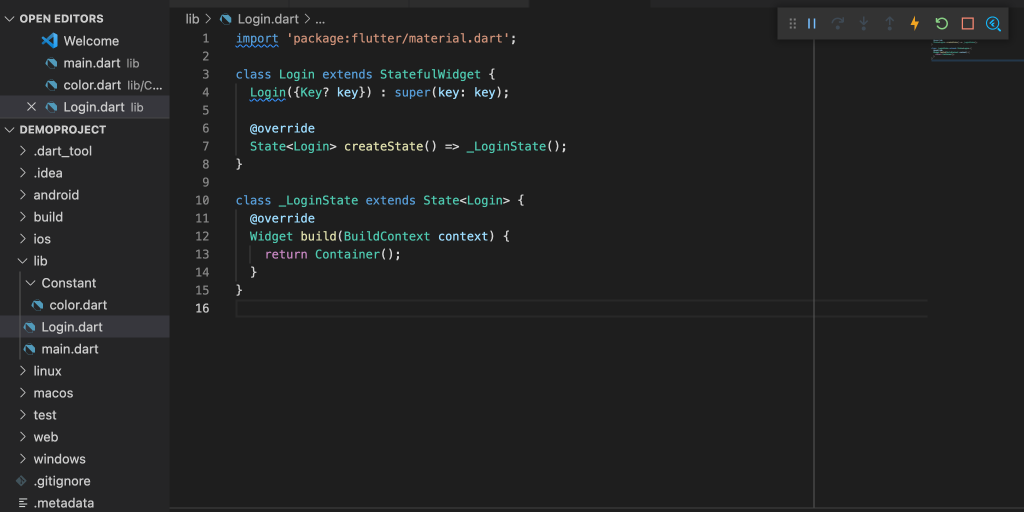
Replace Container() into Scaffold()
The scaffold is use to expand and to fill the available space. That usually means that it will occupy its entire window or device screen.
class Login extends StatefulWidget {
Login({Key? key}) : super(key: key);
@override
State<Login> createState() => _LoginState();
}
class _LoginState extends State<Login> {
@override
Widget build(BuildContext context) {
return Scaffold();
}
}
Background color:
backgroundColor: white,
The color of the Material widget that underlies the entire Scaffold.
Body :
Its the primary content of the scaffold.
SingleChildScrollView ( ) :
A box in which a single widget can be scroll.
SingleChildScrollView widget is useful when you have a single box that will normally be entirely visible.
Column( ) :
Column is to displays its children in a vertical array.
to expand to fill the available vertical space, wrap the child in an Expanded widget.
To arrange the content in center we use ,
example, the crossAxisAlignment is set to CrossAxisAlignment.start, so that the children are left-aligned. The mainAxisSize is set to MainAxisSize.start, so that the column shrinks to fit the children.
Children[ ]
To pass multiple widgets as children in a Column Widget, you need to add each widget as a separate child to the Column Widget. The Column Widget will then arrange the child widgets vertically, one below the other.
Row( )
It creates a horizontal array of children.
to expand to fill the available horizontal space, wrap the child in an Expanded widget.
Alignment Properties: We can align content using mainAxisAlignment and crossAxisAlignment. Row’s mainAxis is horizontal and cross Axis to Row’s main Axis is vertical. We can align children horizontally using MainAxisAlignment and vertically using CrossAxisAlignment in that row.
Flutter uses pubspec.yaml file, located at the root of your project, to identify assets require for the app.
For Example:
flutter:
assets:
- assets/images/logo3.png
- assets/images/
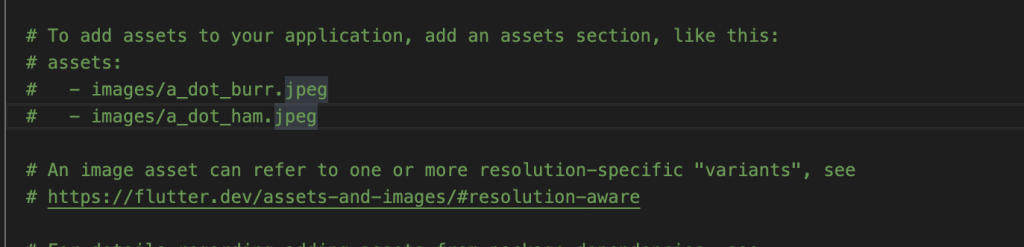
Uncommand the lines and edit what name you have given to the folder of images you have save.
For example,see following codes sippets.

Create file in the name assets Where you can save the image assets needed for the application which includes images,icons,svg,font family.
- If you want to add other assets to your app, like fonts, it is prefer to make another subfolder named images.
- The path should look like assets/images/yourImage.
- Update the assets folder in pubspec.yaml file.
Image.asset(‘assets/images/logo3.png’,)
Centre()
It’s the widget which can centre the element present in it.
Center(
child: Image.asset('assets/images/logo3.png',
height: 150,
fit: BoxFit.cover,
)
),

Text()
The Text widget lets you create a run of styled text within your application.
Textstyle()
Textstyle describing how to format and paint text.
Text can be styled using the property of color,fontSize,fontWeight,fontFamily.
Center(
child: Text(
'Login to your Account',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
color: Colors.black),
),
),
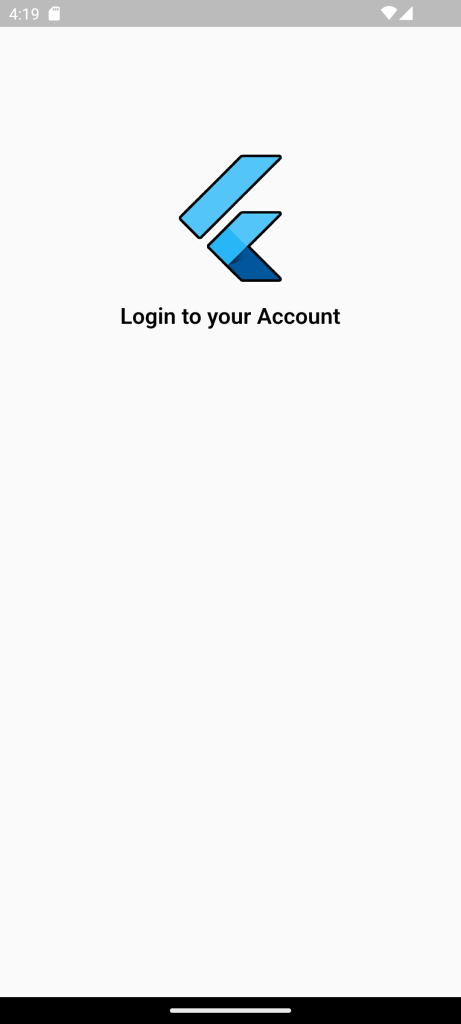
BlankSpace
To make space between the two content use Sizedbox.
SizedBox(
height: 30,
),
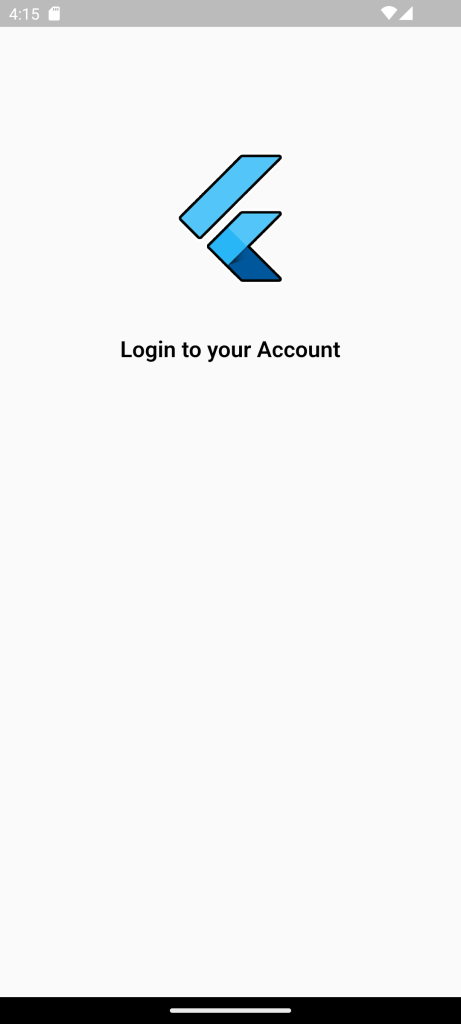
Text(
'Username',
style: TextStyle(
fontSize: 16,
fontWeight: FontWeight.w500,
color: Colors.black),
),
TextField( )
TextField is the most commonly used text input widget.
By default, a TextField is decorated with an underline.
Add a label, icon, inline hint text, and error text by supplying an InputDecoration as the decoration property of the TextField.
TextField(
decoration: InputDecoration(
border: OutlineInputBorder(),
hintText: 'Enter your email',
),
),
TextFormField( )
TextFormField wraps a TextField and integrates it with the enclosing Form. It provides additional functionality, such as validation and integration with other FormField widgets.
InputDecoration( )
The border, labels, icons, and styles used to decorate a Material Design text field.
The TextField and InputDecorator classes use InputDecoration objects to describe their decoration.
- TextField, which is a text input widget that uses an InputDecoration.
- InputDecorator, which is a widget that draws an InputDecoration around an input child widget.
- Decoration and DecoratedBox, for drawing borders and backgrounds around a child widget.
TextEditingController()
The text field updates value and the controller notifies its users. Users can then read the text and selection properties to learn what the user has typed or how the selection has been updated. if you modify the text or selection properties, the text field will be notified and will update itself appropriately.
TextFormField(
keyboardType: TextInputType.emailAddress,
validator: (String? value) {
if (value!.isEmpty) {
return "Please enter username";
}
return null;
},
controller: email,
autocorrect: true,
decoration: InputDecoration(
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: BorderSide(color: Blue, width: 2)),
enabledBorder: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: const BorderSide(color: box),
),
focusedBorder: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: const BorderSide(color: Blue, width: 2),
),
contentPadding:
const EdgeInsets.only(left: 24, top: 14, bottom: 13),
filled: true,
fillColor: Colors.white,
hintText: "Enter your username",
hintStyle: const TextStyle(
color: grey, fontFamily: 'Rubik', fontSize: 14),
prefixIcon: Icon(
Icons.account_circle,
color: Blue,
)),
),
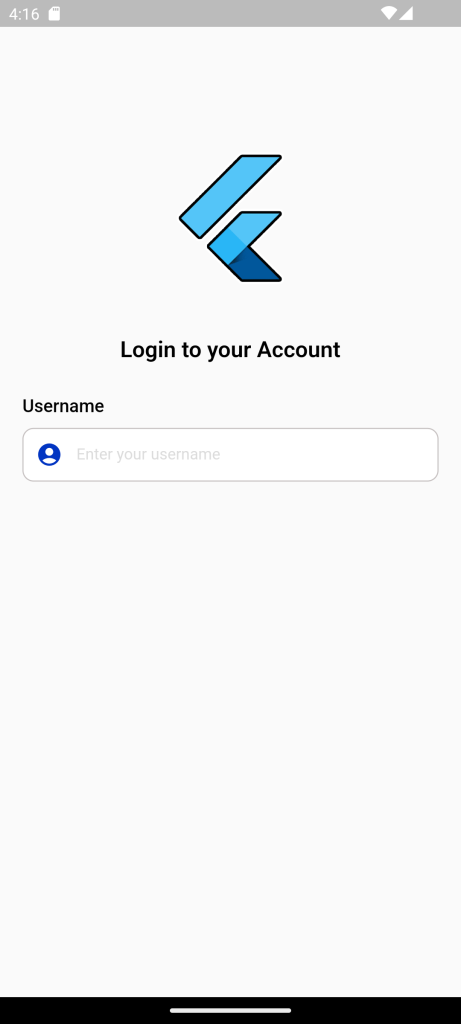
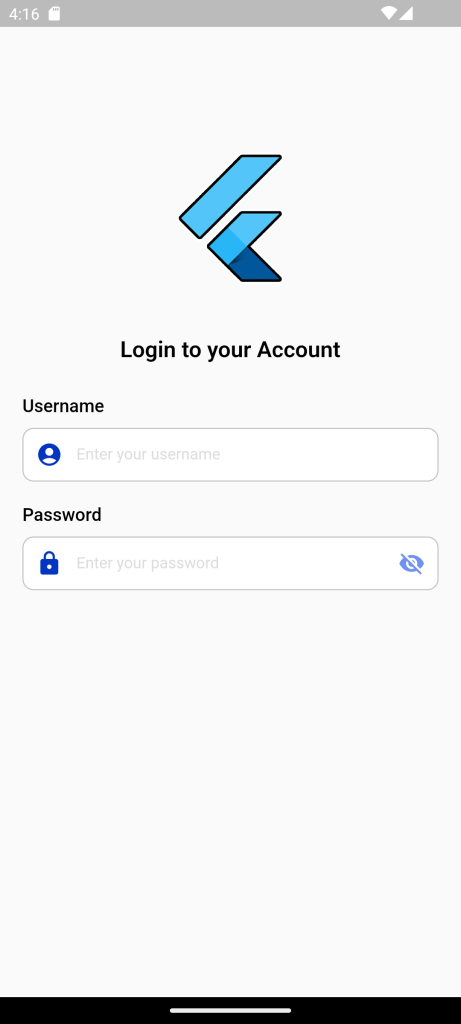
ElevatedButton( )
An elevated button is a label child displayed on a Material widget whose Material elevation increases when the button is pressed.
There are two required parameters. You have to pass a Widget as child, typically a Text widget. You’re also required to pass onPressed callback which is called when the user presses the button.
Sample syntax:
ElevatedButton(
child: Text('Click me'),
onPressed: () {
print('Pressed');
}
)
ElevatedButton(
style: ElevatedButton.styleFrom(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10)),
backgroundColor: Blue,
minimumSize: const Size.fromHeight(44),
),
onPressed: () {
setState(() {
if (formkey.currentState!.validate()) {
isloading = true;
print(isloading);
}
});
},
child: isloading == true
? Container(
width: 24,
height: 24,
padding: const EdgeInsets.all(2.0),
child: const CircularProgressIndicator(
color: Colors.white,
strokeWidth: 3,
),
)
: Text(
'Sign in',
style: TextStyle(
fontSize: 16,
fontFamily: 'IBMPlexSans',
fontWeight: FontWeight.w600,
),
),
),
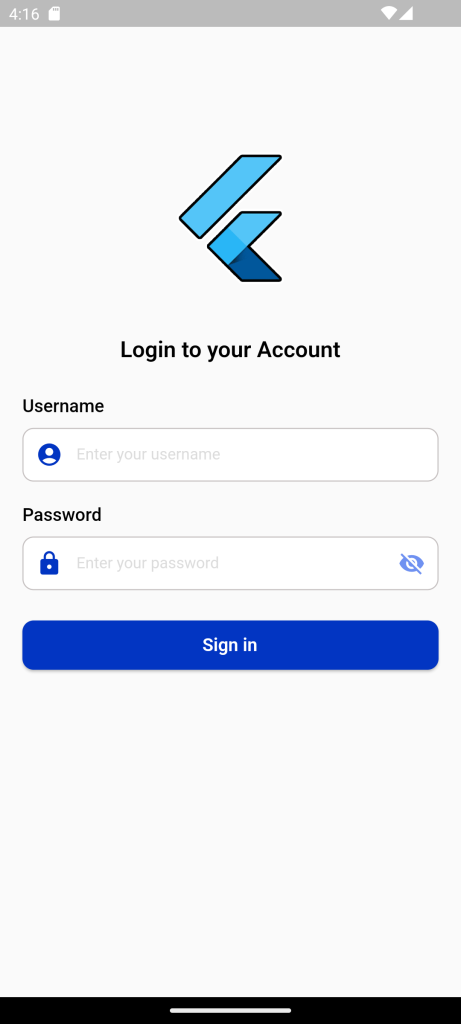
Validation(): Login Page in Flutter
In APP Users to enter information into a text field. For example, you may require users to log in with an email address and password combination.
To make secure and easy to use, check whether the information the user has provided is valid or not. If the user has correctly filled out the information in the form, process the information. If the user submits incorrect information, display a friendly error message letting them know what went wrong.
Follow the steps Whether its Validate or not
- Create a Form with a GlobalKey.
- Add a TextFormFiled with validation logic.
- Create a button to validate and submit the form.
validator: (String? value) {
if (value!.isEmpty) {
return "Please enter username";
}
return null;
},
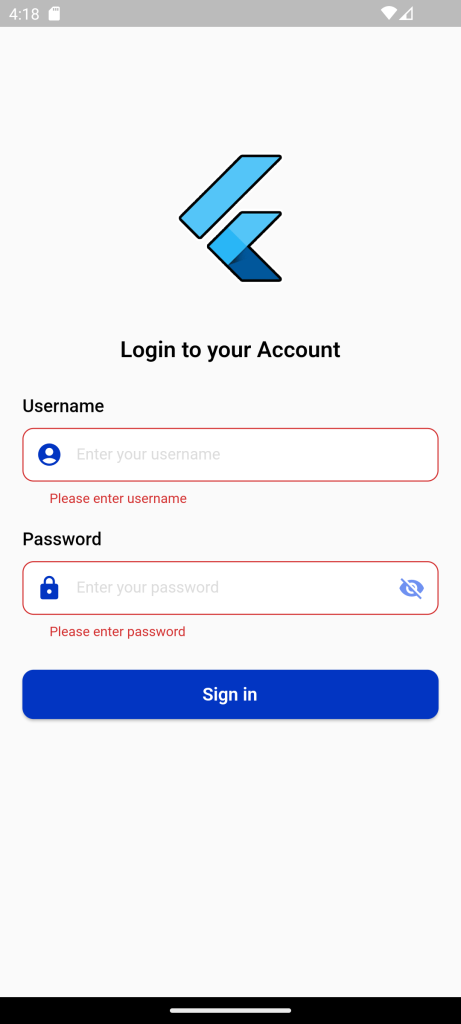
Full Code:Login Page in Flutter
import 'package:demoproject/Constant/color.dart';
import 'package:flutter/material.dart';
class Login extends StatefulWidget {
Login({Key? key}) : super(key: key);
@override
State<Login> createState() => _LoginState();
}
class _LoginState extends State<Login> {
bool isloading = false;
@override
void dispose() {
super.dispose();
}
final GlobalKey<FormState> formkey = GlobalKey<FormState>();
bool _passVisibility = true;
TextEditingController email = TextEditingController();
TextEditingController pass = TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
body: SingleChildScrollView(
child: Form(
key: formkey,
child: Container(
margin: EdgeInsets.only(top: 120),
child: Padding(
padding: const EdgeInsets.only(left: 20, right: 20),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Center(
child: Image.asset(
'assets/images/logo3.png',
height: 150,
fit: BoxFit.cover,
)),
SizedBox(
height: 30,
),
Center(
child: Text(
'Login to your Ac',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
color: Colors.black),
),
),
SizedBox(
height: 30,
),
Text(
'Username',
style: TextStyle(
fontSize: 16,
fontWeight: FontWeight.w500,
color: Colors.black),
),
SizedBox(
height: 10,
),
TextFormField(
keyboardType: TextInputType.emailAddress,
validator: (String? value) {
if (value!.isEmpty) {
return "Please enter username";
}
return null;
},
controller: email,
autocorrect: true,
decoration: InputDecoration(
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: BorderSide(color: Blue, width: 2)),
enabledBorder: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: const BorderSide(color: box),
),
focusedBorder: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: const BorderSide(color: Blue, width: 2),
),
contentPadding:
const EdgeInsets.only(left: 24, top: 14, bottom: 13),
filled: true,
fillColor: Colors.white,
hintText: "Enter your username",
hintStyle: const TextStyle(
color: grey, fontFamily: 'Rubik', fontSize: 14),
prefixIcon: Icon(
Icons.account_circle,
color: Blue,
)),
),
SizedBox(
height: 20,
),
Text(
'Password',
style: TextStyle(
fontSize: 16,
fontWeight: FontWeight.w500,
color: Colors.black),
),
SizedBox(
height: 10,
),
TextFormField(
validator: (String? value) {
if (value!.isEmpty) {
return "Please enter password";
}
return null;
},
controller: pass,
autocorrect: true,
obscureText: _passVisibility,
decoration: InputDecoration(
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: BorderSide(color: red, width: 2)),
enabledBorder: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: const BorderSide(color: box),
),
focusedBorder: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: const BorderSide(color: Blue, width: 2),
),
contentPadding:
const EdgeInsets.only(left: 24, top: 14, bottom: 13),
filled: true,
fillColor: Colors.white,
hintText: 'Enter your password',
hintStyle: const TextStyle(
fontFamily: 'Rubik', color: grey, fontSize: 14),
suffixIcon: IconButton(
color: grey,
icon: _passVisibility
? Icon(
Icons.visibility_off,
color: LiteBlue,
)
: Icon(
Icons.visibility,
color: Blue,
),
onPressed: () {
_passVisibility = !_passVisibility;
setState(() {});
},
),
prefixIcon: Icon(
Icons.lock_rounded,
color: Blue,
)),
),
SizedBox(height: 25),
Center(
child: SizedBox(
width: 200,
child:
ElevatedButton(
style: ElevatedButton.styleFrom(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10)),
backgroundColor: Blue,
minimumSize: const Size.fromHeight(44),
),
onPressed: () {
setState(() {
if (formkey.currentState!.validate()) {
isloading = true;
print(isloading);
}
});
},
child: isloading == true
? Container(
width: 24,
height: 24,
padding: const EdgeInsets.all(2.0),
child: const CircularProgressIndicator(
color: Colors.white,
strokeWidth: 3,
),
)
: Text(
'Sign in',
style: TextStyle(
fontSize: 16,
fontFamily: 'IBMPlexSans',
fontWeight: FontWeight.w600,
),
),
),
),
),
],
),
),
),
)));
}
}
For More : To know about Text Input Box in Flutter
Leave a Reply