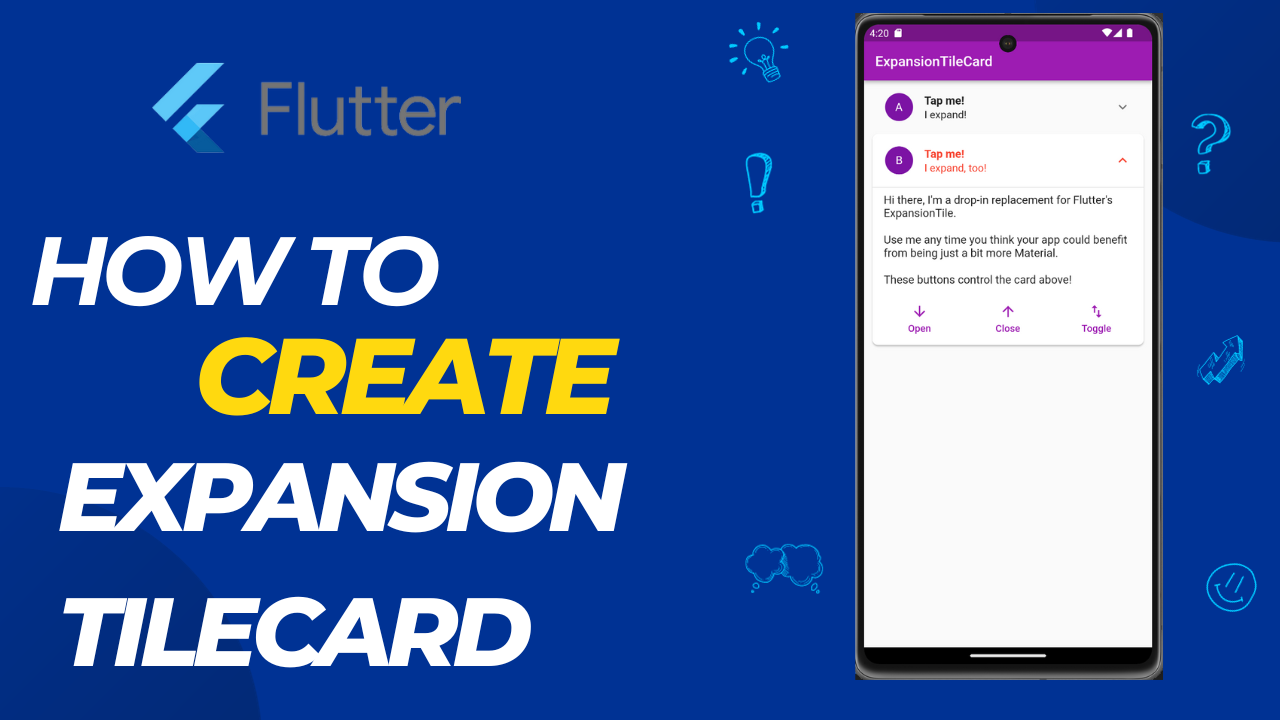
Expansion TileCard in Flutter:An expansion tile in flutter is almost similar to the ListTile which you have already used in list view but the only difference is that the user can expand or collapse the tile to view more details about the tile. You can see the example of an expansion tile in the above image.
ExapnsionTile is mainly use when you want to add some extra details to your ListTile but the details are not requires at the first view. This can view by the user if they want to see it.
Creating a ListView
Before creating an expanding list view let’s just make a basic list view with a list tile. To do that, we just add a ListView.builder() in the body of the scaffold.
class HomeScreen extends StatelessWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(""),
),
body: ListView.builder(
itemCount: 10,
itemBuilder: (context, index) {
return Card(
child: ListTile(
title: Text("User $index"),
),
);
},
));
}
}
Adding ExpansionTile in ListView
Now our basic list view is ready. To make the list item in the list expansible we just need to change the ListTile widget with ExpansionTile.
body: ListView.builder(
itemCount: 10,
itemBuilder: (context, index) {
return Card(
child: ExpansionTile(
title: Text("User $index"),
),
);
},
After changing the ListTile with ExpansionTile you can see there is an arrow at the end of the tile. Now if we click on the tile the tile will expand and will be highlights and the arrow will change its direction.
Properties
The different properties of the Expansion Tile Card are gives below.
- backgroundColor: It defines the color displayed behind the sublist when expands.
- children: It represents the widgets that is display on expansion.
- childrenPadding: It specifies padding for the children.
- collapsedBackgroundColor: When it is not null, it defines the background color when the sublist is collapse.
- collapsedIconColor: It establishes the icon color of the tile’s expansion arrow when the sublist is collapse.
- collapsedTextColor: It defines the color of the tile’s titles when the sublist is collapse.
- expandedAlignment: It specifies the alignment of children, arranged in a column when the tile is expand.
- iconColor: It sets the icon color of the tile’s expansion arrow icon when the sublist is expand.
- initiallyExpanded: It specifies if the list tile is initially expand (true) or collapse (false, the default).
- subtitle: It defines the additional content displayed below the title.
- textColor: It specifies the color of the tile’s titles when the sublist is expand.
- tilePadding: It sets padding for the list tiles.
- title: It determines the primary content of the list item.
Some of these properties will be used when we learn to add the expansion tile card to our application.
We need to follow the below-given steps to add an expansion tile card.
Step 1
Add the “expansion_tile_card” dependency to the “pubspec.yaml” file
Open the pubsec.yaml file, and under the dependencies section, add the dependency of the expansion tile card as shows below.
dependencies:
flutter:
sdk: flutter
expansion_tile_card: ^1.1.0
Step 2
Import the dependency to the “main.dart” file
In the main.dart file uses the below-given code snippet to import the expansion tile card dependency.
import 'package:expansion_tile_card/expansion_tile_card.dart';
Step 3
Create a basic app structure
In the main.dart file create a class MyApp and extend it through a StatelessWidget. Now, we can build the root of the application as shown below.
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'ExpansionTileCard Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'ExpansionTile'),
);
}
}
Step 4
Create a Homepage for the app
We need to build the app’s homepage to display the expansion tile card. To make the homepage, create a Class Homepage and extend it through a StatelessWidget. Now, create an Appbar and a body for the application, as shown below.
class _MyHomePageState extends State<MyHomePage> {
final GlobalKey<ExpansionTileCardState> cardA = new GlobalKey();
final GlobalKey<ExpansionTileCardState> cardB = new GlobalKey();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title!),
),
body:
),
);
}
}
Step 5
Call the ExpansionTileCard in the app’s body and assign content to the Expansion card.
ExpansionTileCard(
key: cardA,
leading: CircleAvatar(child: Text('A')),
title: Text('Tap me!'),
subtitle: Text('I expand!'),
children: <Widget>[
Divider(
thickness: 1.0,
height: 1.0,
),
Align(
alignment: Alignment.centerLeft,
child: Padding(
padding: const EdgeInsets.symmetric(
horizontal: 16.0,
vertical: 8.0,
),
child: Text(
"""Hi there, I'm a drop-in replacement for Flutter's ExpansionTile.
Use me any time you think your app could benefit from being just a bit more Material.
These buttons control the next card down!""",
style: Theme.of(context)
.textTheme
.bodyText2!
.copyWith(fontSize: 16),
),
),
),
Full Code:Expansion TileCard in Flutter
import 'package:flutter/material.dart';
import 'package:expansion_tile_card/expansion_tile_card.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'ExpansionTileCard ',
theme: ThemeData(
primarySwatch: Colors.purple,
),
home: MyHomePage(title: 'ExpansionTileCard '),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key? key, this.title}) : super(key: key);
final String? title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final GlobalKey<ExpansionTileCardState> cardA = new GlobalKey();
final GlobalKey<ExpansionTileCardState> cardB = new GlobalKey();
@override
Widget build(BuildContext context) {
final ButtonStyle TextButtonStyle = TextButton.styleFrom(
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(4.0)),
),
);
return Scaffold(
appBar: AppBar(
title: Text(widget.title!),
),
body: ListView(
children: <Widget>[
Padding(
padding: const EdgeInsets.symmetric(horizontal: 12.0),
child: ExpansionTileCard(
key: cardA,
leading: CircleAvatar(child: Text('A')),
title: Text(
'Tap me!',
style: TextStyle(fontSize: 16, fontWeight: FontWeight.bold),
),
subtitle: Text(
'I expand!',
style: TextStyle(fontSize: 15, fontWeight: FontWeight.w400),
),
children: <Widget>[
Divider(
thickness: 1.0,
height: 1.0,
),
Align(
alignment: Alignment.centerLeft,
child: Padding(
padding: const EdgeInsets.symmetric(
horizontal: 16.0,
vertical: 8.0,
),
child: Text(
"""Hi there, I'm a drop-in replacement for Flutter's ExpansionTile.
Use me any time you think your app could benefit from being just a bit more Material.
These buttons control the next card down!""",
style: Theme.of(context)
.textTheme
.bodyText2!
.copyWith(fontSize: 16),
),
),
),
ButtonBar(
alignment: MainAxisAlignment.spaceAround,
buttonHeight: 52.0,
buttonMinWidth: 90.0,
children: <Widget>[
TextButton(
style: TextButtonStyle,
onPressed: () {
cardB.currentState?.expand();
},
child: Column(
children: <Widget>[
Icon(Icons.arrow_downward),
Padding(
padding: const EdgeInsets.symmetric(vertical: 2.0),
),
Text('Open'),
],
),
),
TextButton(
style: TextButtonStyle,
onPressed: () {
cardB.currentState?.collapse();
},
child: Column(
children: <Widget>[
Icon(Icons.arrow_upward),
Padding(
padding: const EdgeInsets.symmetric(vertical: 2.0),
),
Text('Close'),
],
),
),
TextButton(
style: TextButtonStyle,
onPressed: () {
cardB.currentState?.toggleExpansion();
},
child: Column(
children: <Widget>[
Icon(Icons.swap_vert),
Padding(
padding: const EdgeInsets.symmetric(vertical: 2.0),
),
Text('Toggle'),
],
),
),
],
),
],
),
),
Padding(
padding: const EdgeInsets.symmetric(horizontal: 12.0),
child: ExpansionTileCard(
key: cardB,
expandedTextColor: Colors.red,
leading: CircleAvatar(child: Text('B')),
title: Text(
'Tap me!',
style: TextStyle(fontSize: 16, fontWeight: FontWeight.bold),
),
subtitle: Text(
'I expand, too!',
style: TextStyle(fontSize: 15, fontWeight: FontWeight.w400),
),
children: <Widget>[
Divider(
thickness: 1.0,
height: 1.0,
),
Align(
alignment: Alignment.centerLeft,
child: Padding(
padding: const EdgeInsets.symmetric(
horizontal: 16.0,
vertical: 8.0,
),
child: Text(
"""Hi there, I'm a drop-in replacement for Flutter's ExpansionTile.
Use me any time you think your app could benefit from being just a bit more Material.
These buttons control the card above!""",
style: Theme.of(context)
.textTheme
.bodyText2!
.copyWith(fontSize: 16),
),
),
),
ButtonBar(
alignment: MainAxisAlignment.spaceAround,
buttonHeight: 52.0,
buttonMinWidth: 90.0,
children: <Widget>[
TextButton(
style: TextButtonStyle,
onPressed: () {
cardA.currentState?.expand();
},
child: Column(
children: <Widget>[
Icon(Icons.arrow_downward),
Padding(
padding: const EdgeInsets.symmetric(vertical: 2.0),
),
Text('Open'),
],
),
),
TextButton(
style: TextButtonStyle,
onPressed: () {
cardA.currentState?.collapse();
},
child: Column(
children: <Widget>[
Icon(Icons.arrow_upward),
Padding(
padding: const EdgeInsets.symmetric(vertical: 2.0),
),
Text('Close'),
],
),
),
TextButton(
style: TextButtonStyle,
onPressed: () {
cardA.currentState?.toggleExpansion();
},
child: Column(
children: <Widget>[
Icon(Icons.swap_vert),
Padding(
padding: const EdgeInsets.symmetric(vertical: 2.0),
),
Text('Toggle'),
],
),
),
],
),
],
),
),
],
),
);
}
}
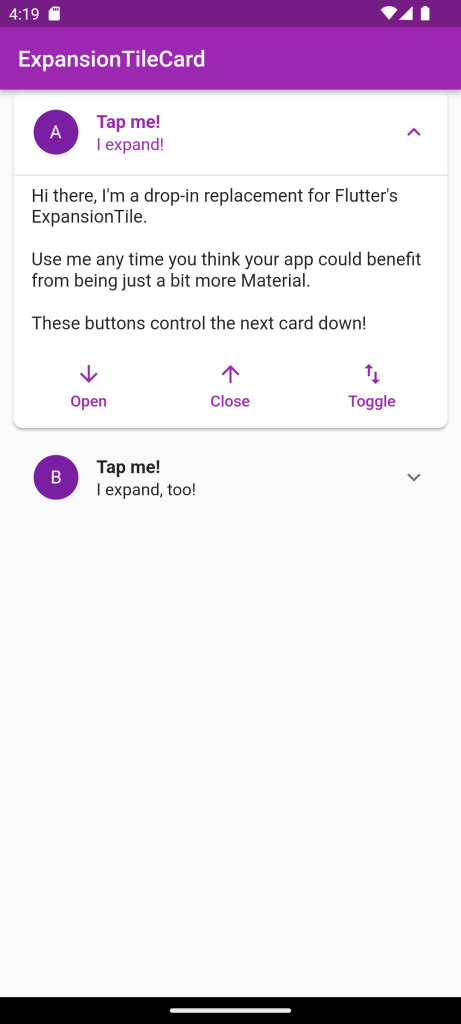
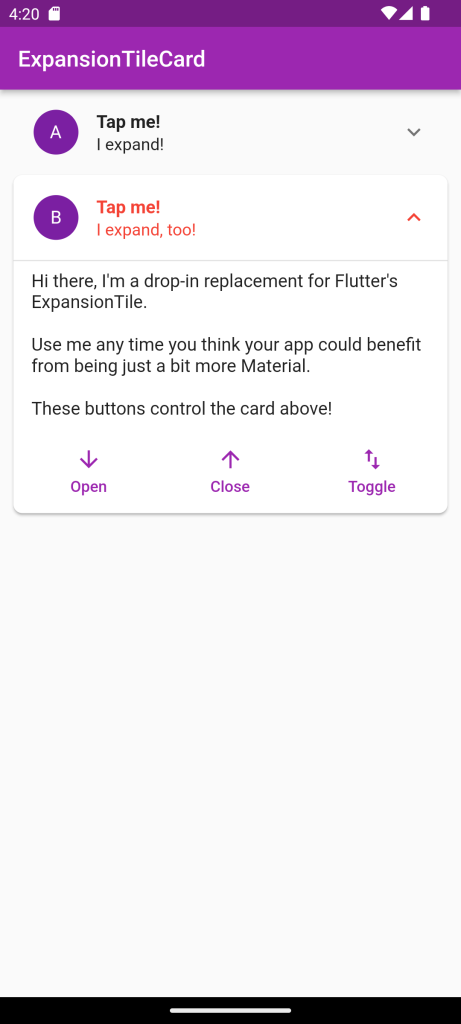
For more: To know about Carousel Slider in Flutter
Leave a Reply