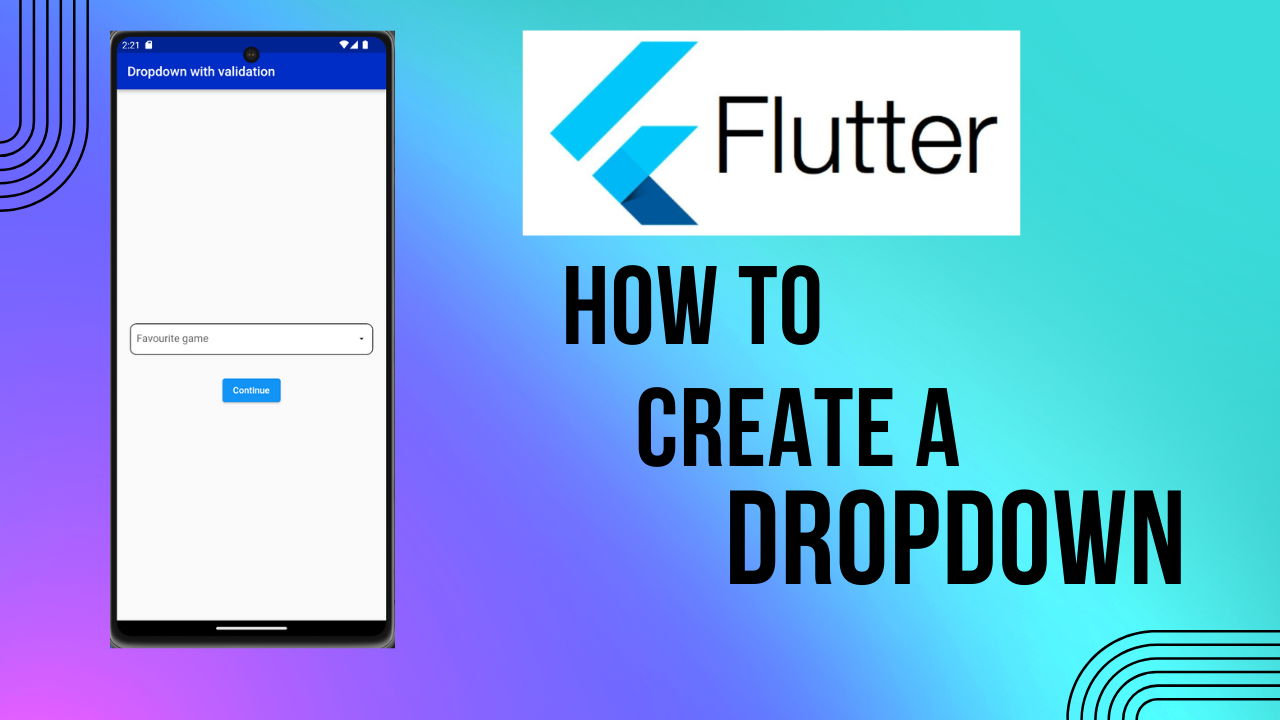
Dropdown in Flutter : Dropdown is a widget that shows a list of items when clicked and shows the selected value.
Flutter provides two types of dropdowns, which are “DropdownButton” and “DropdownButtonFormField” (extra with validator and decoration properties). We will study both widgets by discussing their properties.
How to Create a Dropdown in Flutter?
To create a flutter dropdown, you have to return a DropdownButton widget and use the properties according to your needs.
- Create a list of items to be displayed in the dropdown list.
List<String> gameList = ["Cricket", "Hockey", "Football", "Badminton"];
2.Define a variable that will store the selected item.
var selectedValue;
3.Then pass these data to the DropdownButton.
DropdownButton<String>(
value: selectedValue,
items: gameList.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
onChanged: (String newValue) {
setState(() {
selectedValue = newValue;
});
},
);
Full Code:
import 'package:demoproject/Constant/color.dart';
import 'package:flutter/material.dart';
class DropdownExample extends StatefulWidget {
const DropdownExample({Key? key}) : super(key: key);
@override
State<DropdownExample> createState() => _DropdownExamplwState();
}
class _DropdownExamplwState extends State<DropdownExample> {
var chosenValue;
List<String> languageList = ["Tamil","English","Hindi","Telugu"];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Blue,
title: Text("DropDown"),
titleSpacing: 2,
),
body: Padding(
padding: const EdgeInsets.all(10),
child: Column(
children: [
Padding(
padding: const EdgeInsets.all(10),
child: Container(
padding: const EdgeInsets.all(10),
decoration: BoxDecoration(
color: Colors.blue,
borderRadius: BorderRadius.circular(10)),
child: DropdownButton<String>(
elevation: 1,
underline: SizedBox(),
isExpanded: true,
hint: const Text("Select language"),
iconSize: 30,
iconEnabledColor: Colors.black,
icon: const Icon(
Icons.arrow_drop_down_sharp,
size: 15,
),
value: chosenValue,
style: TextStyle(fontSize: 16, fontWeight: FontWeight.normal),
items: languageList
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
onChanged: (value) {
setState(() {
chosenValue = value;
});
},
alignment: AlignmentDirectional.centerStart,
iconDisabledColor: Colors.purple,
dropdownColor: Colors.blueGrey,
isDense: true,
disabledHint: Container(
color: Colors.deepOrange,
),
selectedItemBuilder: (BuildContext context) {
return languageList.map<Widget>((String item) {
return Container(
alignment: Alignment.centerLeft,
constraints: const BoxConstraints(minWidth: 100),
child: Text(
item,
style: const TextStyle(
color: Colors.black, fontWeight: FontWeight.w600),
),
);
}).toList();
},
),
),
)
],
),
),
);
}
}
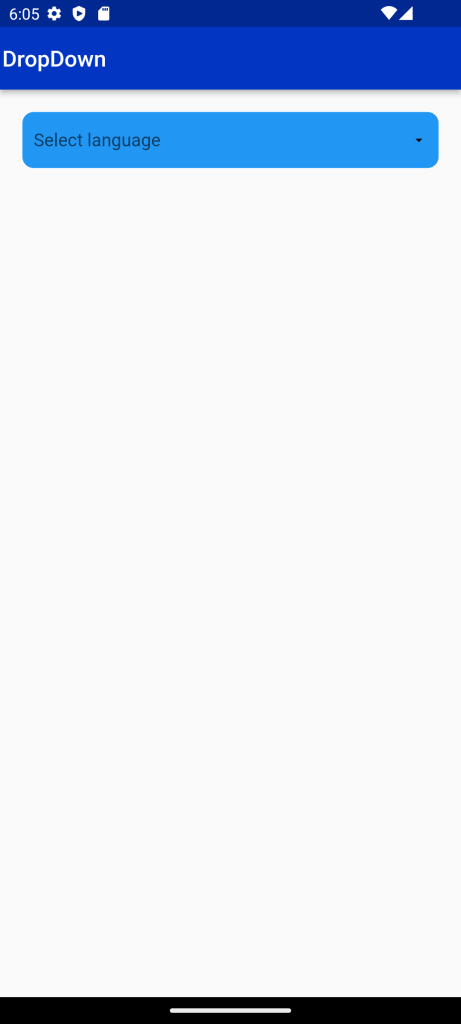
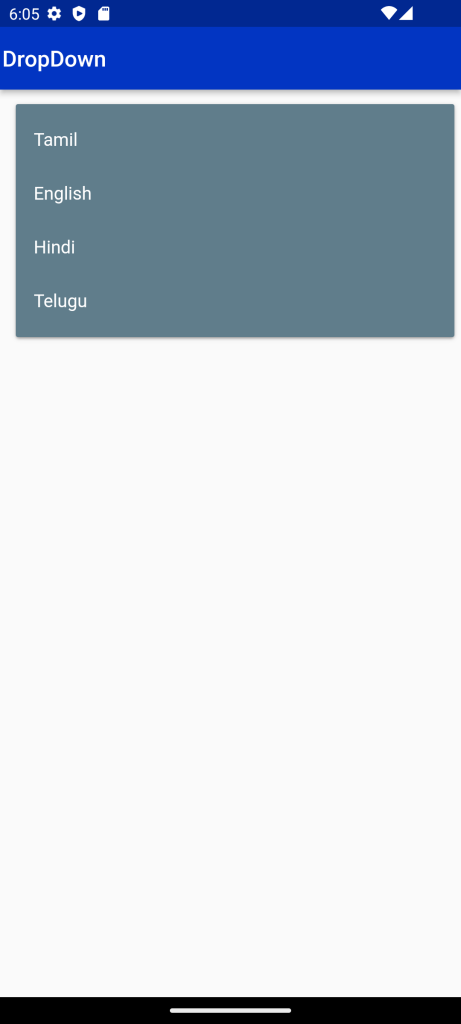
Dropdown with Validation
It means we have to validate our flutter dropdown, i.e., make it compulsory to select an item from the list; otherwise, it will show an error.
In this case, either we can show only a red outer border or we can display some text as shown below, or both.
So, for this we have to keep two things in mind –
- Wrap the flutter dropdown widget with the “Form” widget and we have to pass a global key in the Form widget.
- For this we have to use “DropdownButtonFormField” instead of “DropdownButton”.
Steps to follow:
- First, we have to create a key of type GlobalKey inside the class
final formKey = GlobalKey<FormState>();
2.Then wrap the “DropdownButtonFormField” with Form widget and pass the global key defined above in the key property of the Form widget.
Form(
key: formKey,
child: DropdownButtonFormField<String>()
)
3.Inside DropdownButtonFormField, it is mandatory to pass the validator property to show the error text by checking null and empty conditions.Also to show the red color border, you have to pass decoration property and define the error border inside it where you can chaitsit’s color, width, and borderRadius.
Form(
key: formKey,
child: DropdownButtonFormField<String>(
decoration: InputDecoration(
errorBorder: OutlineInputBorder(
borderSide: const BorderSide(width: 1,color: Colors.red),
borderRadius: BorderRadius.circular(10)),
),
validator: (value) {
if (value == null || value.isEmpty) {
return 'Select some role';
} else {
return null;
}
},
)
)
4.Then inside the Button widget, you have to validate using GlobalKey variable by pressing the button. We have to validate the current state of the key and return the validator defined in the flutter dropdown button if not valid.
Full Code: Dropdown in Flutter
import 'package:flutter/material.dart';
class DropdownExample extends StatefulWidget {
const DropdownExample({Key? key}) : super(key: key);
@override
State<DropdownExample> createState() => _DropdownExampleState();
}
class _DropdownExampleState extends State<DropdownExample> {
final formKey = GlobalKey<FormState>();
var chosenValue;
List<String> gameList = ["Cricket", "Hockey", "Football", "Badminton"];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Blue,
title: const Text("Dropdown with validation"),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Padding(
padding: const EdgeInsets.symmetric(horizontal: 20.0, vertical: 30),
child: Form(
key: formKey,
child: DropdownButtonFormField<String>(
decoration: InputDecoration(
fillColor: Colors.white,
filled: true,
contentPadding: const EdgeInsets.only(right: 10, left: 10),
border: const OutlineInputBorder(
borderSide: BorderSide(color: Colors.black, width: 1.0),
borderRadius: BorderRadius.all(
Radius.circular(10.0),
),
),
focusedBorder: const OutlineInputBorder(
borderSide: BorderSide(color: Colors.black, width: 1.0),
borderRadius: BorderRadius.all(
Radius.circular(10.0),
),
),
enabledBorder: const OutlineInputBorder(
borderSide: BorderSide(color: Colors.black, width: 1.0),
borderRadius: BorderRadius.all(
Radius.circular(10.0),
),
),
errorBorder: OutlineInputBorder(
borderSide: const BorderSide(width: 1, color: Colors.red),
borderRadius: BorderRadius.circular(10)),
),
elevation: 1,
validator: (value) {
if (value == null || value.isEmpty) {
return 'Select game';
} else {
return null;
}
},
isExpanded: true,
hint: const Text("Favourite game"),
iconSize: 30,
iconEnabledColor: Colors.black,
icon: const Icon(
Icons.arrow_drop_down_sharp,
size: 15,
),
value: chosenValue,
items: gameList.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
onChanged: (value) {
setState(() {
chosenValue = value;
});
},
),
),
),
ElevatedButton(
onPressed: () {
final isValid = formKey.currentState!.validate();
if (!isValid) return;
},
child: const Text("Continue"))
],
),
);
}
}
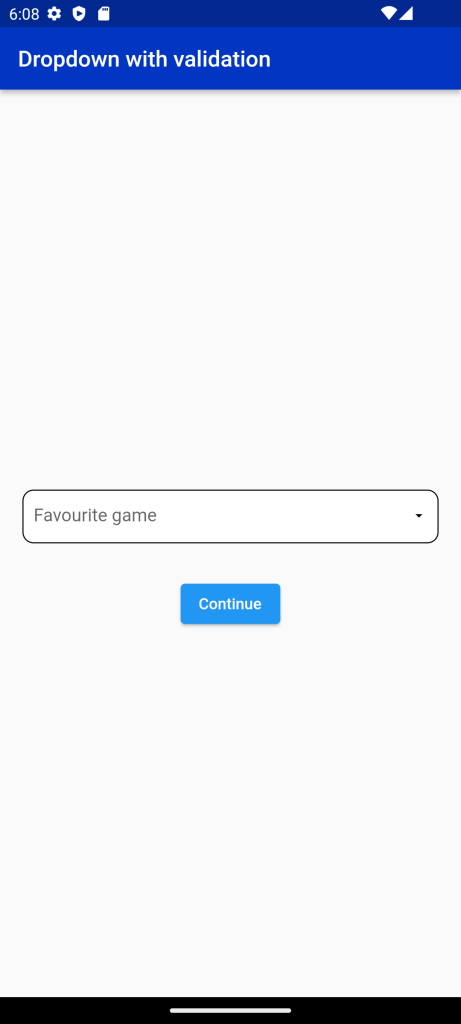
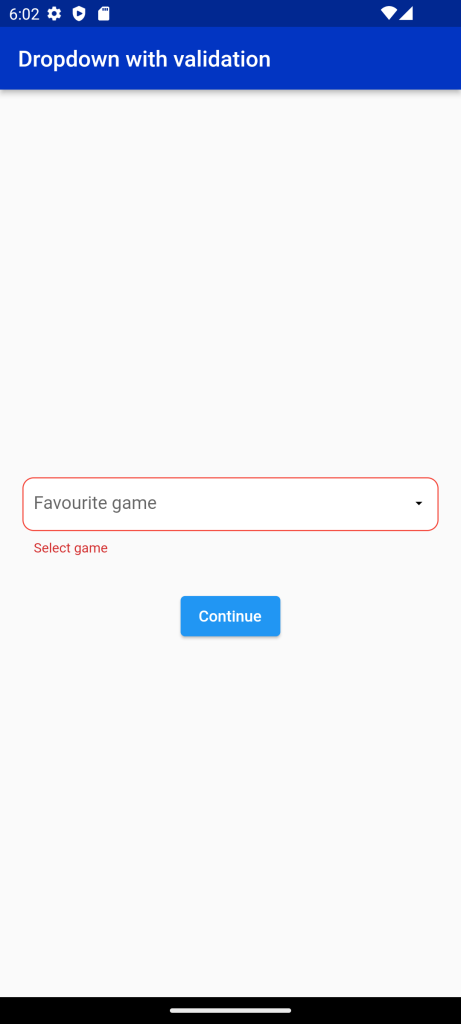
For more: To know about Popup Menu in Flutter
Leave a Reply