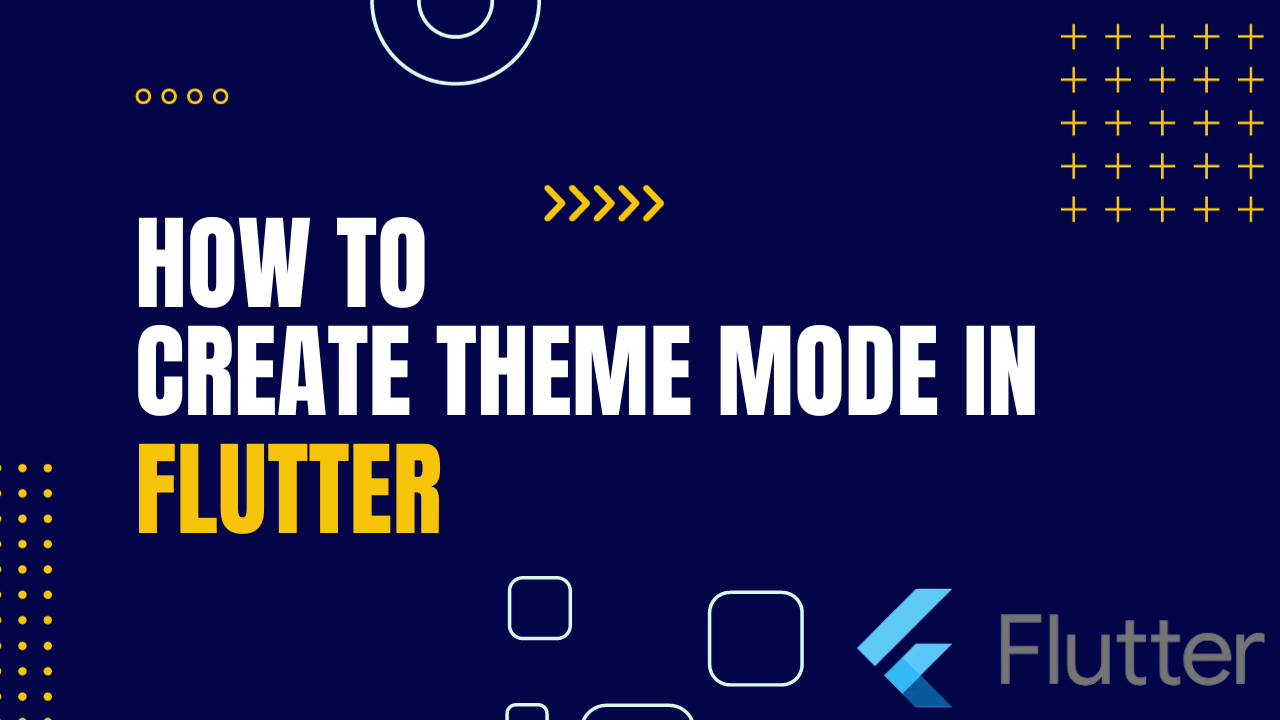
Flutter Theme Mode using Provider:Nowadays almost all the mobile application uses a dark theme, for example, Instagram, Whatsapp, Youtube, etc. It’s easy to implement in Flutter,
we will be learning how to implement the dark theme and light in our app using the provider
package
As we all now Dark theme is trending and most of the popular app has the feature to turn into the dark mode.
Dark Mode:
The dark mode is characterized by darker backgrounds and lighter text/icons. It’s designed to reduce the amount of light emitted by screens, which can be beneficial in various scenarios:
- Reduced Eye Strain: Dark mode can be easier on the eyes, especially in low-light environments. The lower brightness of dark backgrounds can help reduce eye strain and visual fatigue.
- Better for OLED Displays: OLED displays can individually turn off pixels to display true black. In dark mode, using black backgrounds can result in energy savings and better contrast.
- Preserving Night Vision: When using devices in the dark, such as during bedtime, the dark mode can help preserve your night vision and make it easier to transition to a dark environment.
- Energy Efficiency: For devices with OLED or AMOLED screens, the dark mode can save battery life since individual pixels can be turned off, using less power.
- Aesthetics: Many users find dark mode visually appealing and modern. It can also reduce the glare of a bright screen, creating a more immersive experience.
Light Mode:
Light mode is the traditional color scheme with light backgrounds and dark text/icons. It offers its own set of advantages:
- Readability: Light mode often provides better readability, especially in well-lit environments. The high contrast between dark text and light backgrounds can make text stand out more clearly.
- Familiarity: Light mode is the default mode in most applications and websites. Users who are accustomed to this mode may find it more comfortable and familiar.
- Accessibility: Light mode is generally more accessible for individuals with certain visual impairments, as the high contrast between text and background can enhance legibility.
- Consistency with Branding: For some apps and websites, the light mode may align better with their branding and design aesthetics, providing a cleaner and more professional look.
Implementation
Step 1: Add the dependencies
Add dependencies to pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
shared_preferences:
provider: ^6.1.1
switcher_button: ^0.0.4
Step 2: Create folders and dart files inside the lib folder like Shared Preferences, Pages, and Theam, and inside these folders, we create the dart files for better understanding and good practice for the projects.
import 'package:shared_preferences/shared_preferences.dart';
class TheamSharredPreferences{
static const PREF_KEY = "preferences";
setTheam(bool value) async{
SharedPreferences sharedPreferences = await SharedPreferences.getInstance();
sharedPreferences.setBool(PREF_KEY, value);
}
getTheam() async{
SharedPreferences sharedPreferences = await SharedPreferences.getInstance();
return sharedPreferences.getBool(PREF_KEY) ?? false;
}
}
Step 3: Create a model class for provider
import 'package:demo1/Constant/theme.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class TheamModal extends ChangeNotifier {
late bool _isDark;
late ThemeSharredPreferences theamSharredPreferences;
bool get isDark => _isDark;
TheamModal() {
_isDark = false;
theamSharredPreferences = ThemeSharredPreferences();
getTheamPreferences();
}
set isDark(bool value) {
_isDark = value;
theamSharredPreferences.setTheme(value);
notifyListeners();
}
getTheamPreferences() async {
_isDark = await theamSharredPreferences.getTheme();
notifyListeners();
}
}
We are accessing the preference value through the provider
so whenever there is any change the notifyListeners()
UI will be updated if we have attached the provider to the screen.
Step 4: Add the provider to Material app
void main() {
runApp(MyApp());
}
import 'package:demo1/Constant/mode.dart';
import 'package:demo1/mode.dart';
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return ChangeNotifierProvider(
create: (_) => TheamModal(),
child: Consumer(
builder: (context, TheamModal theamModal, child) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: "Example For Dark and Light Mode",
theme: theamModal.isDark ? ThemeData.dark() : ThemeData.light(),
home: HomePage(),
);
},
));
}
}
Step 5:Flutter Theme Mode using Provider
Homepage.dart
import 'package:demo1/Constant/mode.dart';
import 'package:flutter/material.dart';
import 'package:flutter/cupertino.dart';
import 'package:provider/provider.dart';
import 'package:switcher_button/switcher_button.dart';
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return Consumer(
builder: (context, TheamModal theamNotifier, child) {
return Scaffold(
appBar: AppBar(
title: Text(theamNotifier.isDark ? "Dark Theme" : "Light Theme"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
SwitcherButton(
value: theamNotifier.isDark ? false : true,
onChange: (value) {
theamNotifier.isDark
? theamNotifier.isDark = false
: theamNotifier.isDark = true;
},
),
SizedBox(
height: 40,
),
Text("This is a demo for Theme Swicher"),
],
),
),
);
},
);
}
}
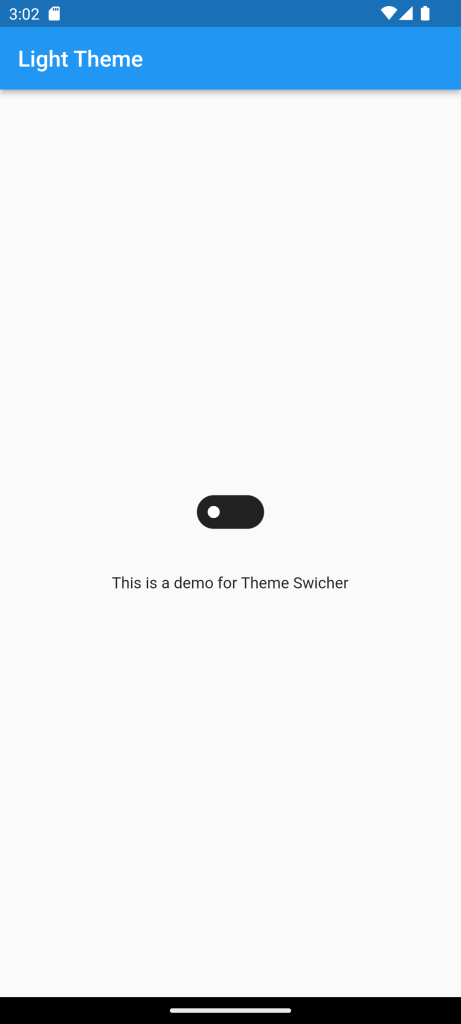
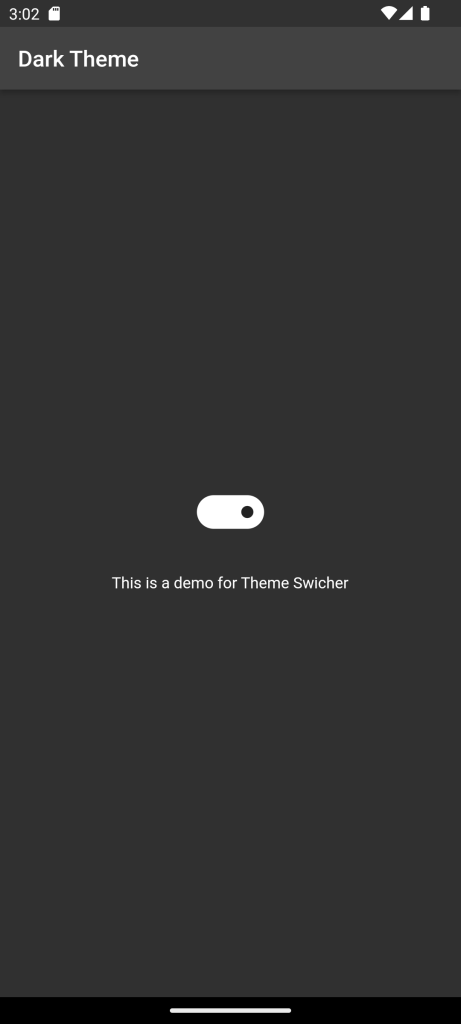
For More: State Management System Bloc Shopping Cart
Leave a Reply