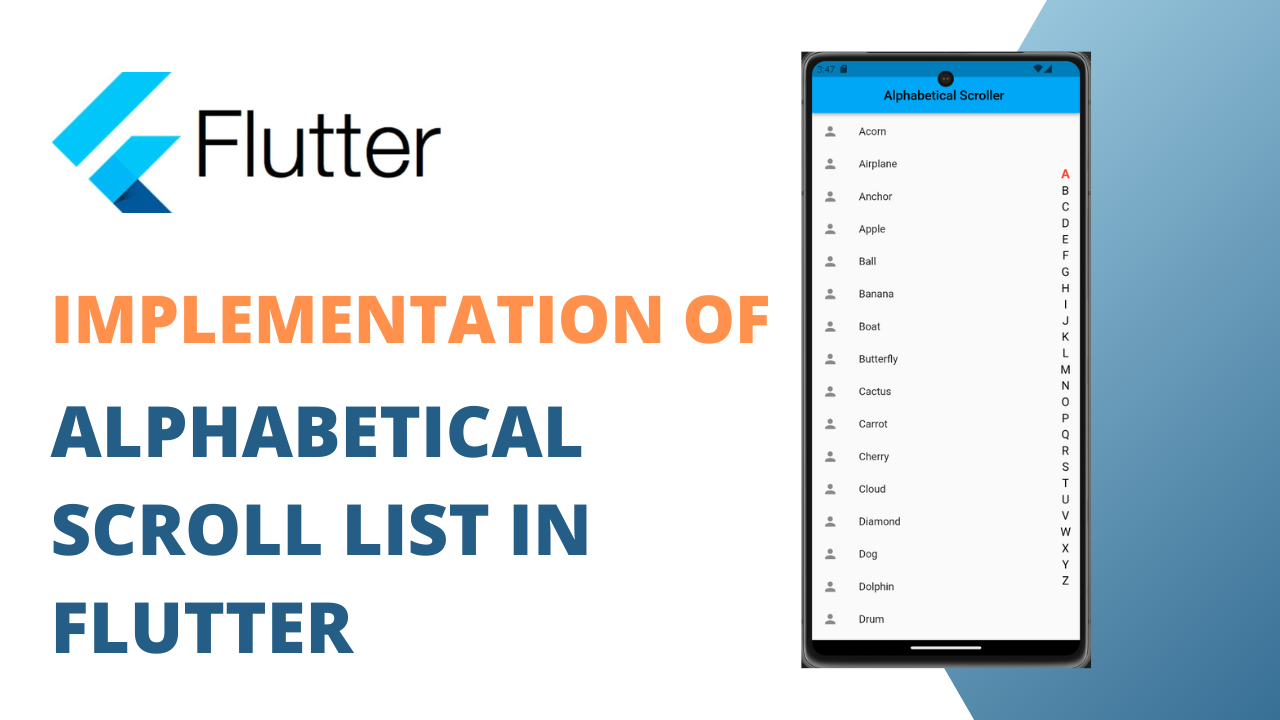
Alphabetical scroll list in flutter:An Alphabetical Scroll List also known as A-Z Scroll List, is the most commonly use in mobile applications that help users to quickly navigate to any large set of list in an alphabetical order.
Here are some common places where an Aplhabetical Scroll List can be used:
- Contact List.
- Music & Video Playlist.
- Address Books.
- Product Lists.
- Country or City List.
An Alphabetical Scroll List is versatile UI element that ca be applied in many use case like contact list, music, video, address, country and many more for efficient navigation through the large dataset.
Features
- Responsive on all screens of different sizes and runs on all Flutter-supported platforms
- show your own widget(
overlayWidget
) when the pointer is in focus with Screen - Align the alphabet list on the left or right
- Tap or drag to scroll to a particular Alphabet.
Implement alphabetical scroll list in flutter
In Flutter to create an alphabetical listview which can be scrollable using easily A-Z Alphabet. We will be using a dependency/library called alphabetical scroll view that allow scrolling to a specific items in the list for easy navigation.
In this guide, we will learn how to implement the Alphabetical Scroll in Flutter by using the external package name alphabet_scroll_view .
A Scrollable ListView Widget with the dynamic vertical Alphabet List on the Side which you can drag and tap to scroll to the first item starting with that letter in the list.
Install Library into flutter project
Open pubspec.yaml file and under dependencies section add:
dependencies:
alphabet_scroll_view: ^0.3.2
After adding the dependencies hit pub get button or enter “flutter pub get” in terminal to download the package into your flutter project as external packages.
import 'package:alphabet_scroll_view/alphabet_scroll_view.dart';
Let’s take an example of how we can implement the Alphabetical Scroll (A-Z) in our flutter application.
Full Code:
import 'package:flutter/material.dart';
import 'package:alphabet_scroll_view/alphabet_scroll_view.dart';
class AlphabeticalScrollerWidegt extends StatefulWidget {
const AlphabeticalScrollerWidegt({Key? key}) : super(key: key);
@override
State<AlphabeticalScrollerWidegt> createState() =>
_AlphabeticalScrollerWidegtState();
}
class _AlphabeticalScrollerWidegtState
extends State<AlphabeticalScrollerWidegt> {
List<String> list = [
"Apple",
"Banana",
"Cherry",
"Dog",
"Elephant",
"Frog",
"Grape",
"Hat",
"Igloo",
"Jump",
"Kite",
"Lemon",
"Moon",
"Nest",
"Orange",
"Penguin",
"Quilt",
"Rabbit",
"Sun",
"Tiger",
"Umbrella",
"Violin",
"Water",
"Xylophone",
"Yellow",
"Zebra",
"Anchor",
"Butterfly",
"Carrot",
"Drum",
"Elephant",
"Feather",
"Guitar",
"Hammer",
"Ice",
"Jelly",
"Kangaroo",
"Lighthouse",
"Mountain",
"Nut",
"Ocean",
"Pencil",
"Quill",
"Rainbow",
"Sailboat",
"Tree",
"Unicorn",
"Volcano",
"Watermelon",
"X-ray",
"Yo-yo",
"Zeppelin",
"Airplane",
"Ball",
"Cloud",
"Dolphin",
"Easel",
"Fire",
"Globe",
"Hamburger",
"Ice Cream",
"Jigsaw",
"Key",
"Lollipop",
"Mushroom",
"Notebook",
"Owl",
"Pizza",
"Quiver",
"Rose",
"Star",
"Turtle",
"Ufo",
"Vase",
"Windmill",
"Xbox",
"Yarn",
"Zipper",
"Acorn",
"Boat",
"Cactus",
"Diamond",
"Ferris Wheel",
"Giraffe",
"Honey",
"Island",
"Jacket",
"Kettle",
"Lantern",
"Map",
"Nutmeg",
"Oar",
"Popcorn",
"Quasar",
"Ruler",
"Shoes",
"Telescope",
"Umpire",
"Valley"
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Alphabetical Scroller '),
centerTitle: true,
),
body: Column(
children: [
Expanded(
child: AlphabetScrollView(
list: list.map((e) => AlphaModel(e)).toList(),
// isAlphabetsFiltered: false,
alignment: LetterAlignment.right,
itemExtent: 50,
unselectedTextStyle: const TextStyle(
fontSize: 18,
fontWeight: FontWeight.normal,
color: Colors.black),
selectedTextStyle: const TextStyle(
fontSize: 20, fontWeight: FontWeight.bold, color: Colors.red),
itemBuilder: (_, index, string) {
return Padding(
padding: const EdgeInsets.only(right: 20),
child: ListTile(
title: Text(string),
leading: const Icon(Icons.person),
),
);
},
),
)
],
),
);
}
}
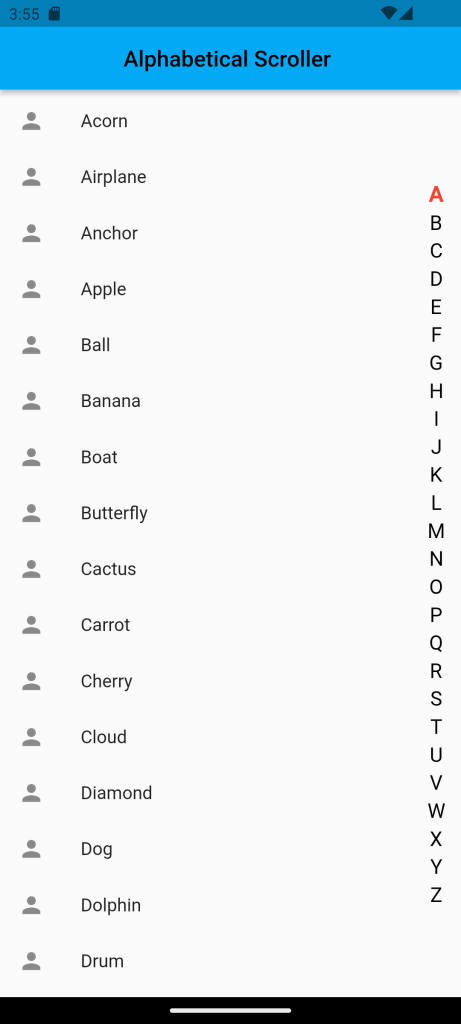
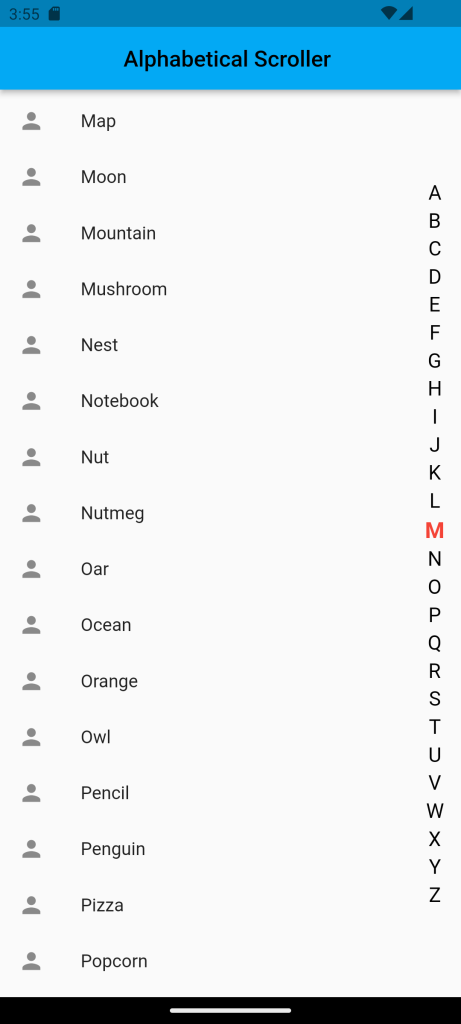
For More : To Know about Flutter Custom Login Page
Leave a Reply