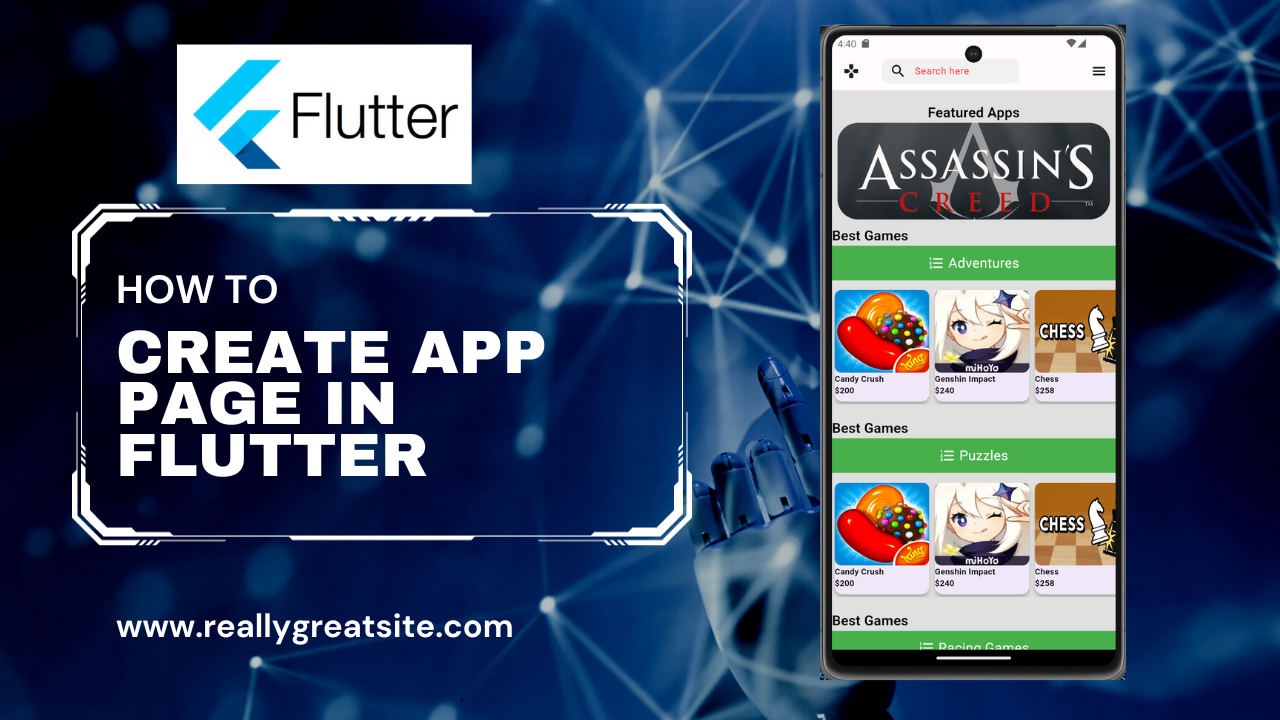
App Download Page UI : Creating a Flutter app for Downloading multiple apps for user need.In day to day life millions of applications are downloads in playstore,applestore.
using the following steps gives below
ListView is a very important widget in a flutter. It is use to create the list of children But when we want to create a list recursively without writing code again and again then ListView.builder is used instead of ListView. ListView.builder creates a scrollable, linear array of widgets.
ListView.builder
is a powerful Flutter widget use for efficiently creating scrollable lists or grids of items. It’s particularly useful when dealing with a large number of items because it creates items on-demand as the user scrolls, reducing memory usage and improving performance. Here’s an explanation of its main components:
- itemCount: This property defines the number of items in the list. It specifies how many times the
itemBuilder
function will be call. - itemBuilder: This callback function is call for each item in the list. It takes two arguments:
BuildContext
andint index
. You’re responsible for returning the widget for the item at the given index. - shrinkWrap: If set to
true
, theListView
will take up only as much vertical space as needed to display its children. It can be useful when theListView
is placed inside another scrollable widget. - physics: Defines the scrolling behavior. You can choose from various options like
BouncingScrollPhysics
,ClampingScrollPhysics
, and more, depending on how you want the scrolling to behave. - scrollDirection: Specifies the direction in which the list should scroll. It can be either
Axis.vertical
(default) for a vertical list orAxis.horizontal
for a horizontal list.
final List<CateogryItem> _cateogry = [
CateogryItem(
title: "Adventures",
name: "Candy Crush",
price: "\$200",
comparePrice: "\$198",
image: 'assets/games/game1.png',
), CateogryItem(
title: "Puzzles",
name: "Genshin Impact",
price: "\$240",
comparePrice: "\$300",
image: 'assets/games/game2.png',
),
CateogryItem(
title: "Racing Games",
name: "Chess",
price: "\$258",
comparePrice: "\$289",
image: 'assets/games/game3.png',
),
CateogryItem(
title: "Action Games",
name: "Creed",
price: "\$240",
comparePrice: "\$300",
image: 'assets/games/game4.png',
),
CateogryItem(
title: "Zombie Games",
name: "Cyberpunk",
price: "\$200",
comparePrice: "\$198",
image: 'assets/games/game5.png',
),
CateogryItem(
title: "Mind Games",
name: "Super Candy",
price: "\$240",
comparePrice: "\$300",
image: 'assets/games/game1.png',
),
];
AppBar(
leading: IconButton(
onPressed: () {},
icon: const Icon(
Icons.games,
)),
title: SizedBox(
height: 35,
width: 200,
child: TextField(
style: const TextStyle(
color: Colors.grey,
fontSize: 20,
fontWeight: FontWeight.w400,
letterSpacing: 0.5,
),
//onChanged: _handleSearch,
decoration: InputDecoration(
filled: true,
fillColor: const Color(0xfff1f1f1),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: BorderSide.none,
),
hintText: "Search here",
hintStyle: const TextStyle(
color: Colors.red,
fontSize: 14,
fontWeight: FontWeight.w400,
letterSpacing: 0.5,
),
prefixIcon: const Icon(Icons.search),
prefixIconColor: Colors.black,
),
),
),
actions: [
IconButton(
onPressed: () {},
icon: const Icon(
Icons.menu,
color: Colors.black,
))
],
),
Full code:App Download Page UI
import 'package:flutter/material.dart';
class Product extends StatefulWidget {
const Product({super.key});
@override
State<Product> createState() => _ProductState();
}
class CateogryItem {
String title;
String price;
String comparePrice;
String image;
String name;
CateogryItem({
required this.title,
required this.price,
required this.comparePrice,
required this.image,
required this.name,
});
}
class _ProductState extends State<Product> {
final List<CateogryItem> _cateogry = [
CateogryItem(
title: "Adventures",
name: "Candy Crush",
price: "\$200",
comparePrice: "\$198",
image: 'assets/games/game1.png',
),
CateogryItem(
title: "Puzzles",
name: "Genshin Impact",
price: "\$240",
comparePrice: "\$300",
image: 'assets/games/game2.png',
),
CateogryItem(
title: "Racing Games",
name: "Chess",
price: "\$258",
comparePrice: "\$289",
image: 'assets/games/game3.png',
),
CateogryItem(
title: "Action Games",
name: "Creed",
price: "\$240",
comparePrice: "\$300",
image: 'assets/games/game4.png',
),
CateogryItem(
title: "Zombie Games",
name: "Cyberpunk",
price: "\$200",
comparePrice: "\$198",
image: 'assets/games/game5.png',
),
CateogryItem(
title: "Mind Games",
name: "Super Candy",
price: "\$240",
comparePrice: "\$300",
image: 'assets/games/game1.png',
),
];
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.grey.shade300,
appBar: AppBar(
leading: IconButton(
onPressed: () {},
icon: const Icon(
Icons.games,
)),
title: SizedBox(
height: 35,
width: 200,
child: TextField(
style: const TextStyle(
color: Colors.grey,
fontSize: 20,
fontWeight: FontWeight.w400,
letterSpacing: 0.5,
),
//onChanged: _handleSearch,
decoration: InputDecoration(
filled: true,
fillColor: const Color(0xfff1f1f1),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(10),
borderSide: BorderSide.none,
),
hintText: "Search here",
hintStyle: const TextStyle(
color: Colors.red,
fontSize: 14,
fontWeight: FontWeight.w400,
letterSpacing: 0.5,
),
prefixIcon: const Icon(Icons.search),
prefixIconColor: Colors.black,
),
),
),
actions: [
IconButton(
onPressed: () {},
icon: const Icon(
Icons.menu,
color: Colors.black,
))
],
),
body: GestureDetector(
behavior: HitTestBehavior.opaque,
onPanDown: (detail) {
print(detail);
FocusScope.of(context).requestFocus(FocusNode());
},
child: ListView(
children: <Widget>[
const SizedBox(height: 10.0),
Padding(
padding: const EdgeInsets.all(8.0),
child: Container(
height: 170.0,
// color: Colors.blue,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text("Featured Apps",
style: const TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
color: Colors.black)),
ClipRRect(
borderRadius: BorderRadius.all(Radius.circular(30)),
child: Image(
image: AssetImage('assets/games/game4.png'),
fit: BoxFit.cover,
height: 140,
width: double.infinity,
),
),
],
)
// const Row(
// mainAxisAlignment: MainAxisAlignment.start,
// children: <Widget>[
// //Icon(Icons.hourglass_empty, color: Colors.white, size: 30.0),
// Padding(padding: EdgeInsets.only(right: 5.0)),
// // Text('TOP5',
// // style: TextStyle(color: Colors.white, fontSize: 23.0)),
// Image(
// image: AssetImage('assets/games/game4.png'),
// fit: BoxFit.cover,
// )
// ],
// ),
),
),
// const SizedBox(height: 10.0),
ListView.builder(
shrinkWrap: true,
itemCount: 5,
physics: const NeverScrollableScrollPhysics(),
itemBuilder: (context, index) {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text("Best Games",
style: const TextStyle(
fontWeight: FontWeight.w600,
fontSize: 20.0,
color: Colors.black)),
Container(
height: 50.0,
color: Colors.green,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Icon(Icons.format_list_numbered,
color: Colors.white),
const Padding(padding: EdgeInsets.only(right: 5.0)),
Text(_cateogry[index].title,
style: const TextStyle(
fontSize: 20.0, color: Colors.white)),
],
),
),
const SizedBox(height: 10.0),
Container(
height: 170.0,
child: ListView.builder(
shrinkWrap: true,
scrollDirection: Axis.horizontal,
itemCount: _cateogry.length,
itemBuilder: (context, index) {
return GestureDetector(
child: Card(
elevation: 5.0,
child: Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width / 3,
alignment: Alignment.center,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
ClipRRect(
borderRadius:
BorderRadius.all(Radius.circular(10)),
child: Image.asset(
_cateogry[index].image,
height: 120,
width: double.infinity,
fit: BoxFit.fill,
),
),
// Text('Item $index'),
Text(_cateogry[index].name,
style: const TextStyle(
fontSize: 12,
fontWeight: FontWeight.w600,
color: Colors.black)),
Text(_cateogry[index].price,
style: const TextStyle(
fontSize: 12,
fontWeight: FontWeight.w600,
color: Colors.black)),
],
),
),
),
onTap: () {
print(123);
},
);
},
),
),
const SizedBox(height: 20.0),
],
);
},
),
],
),
),
);
}
}
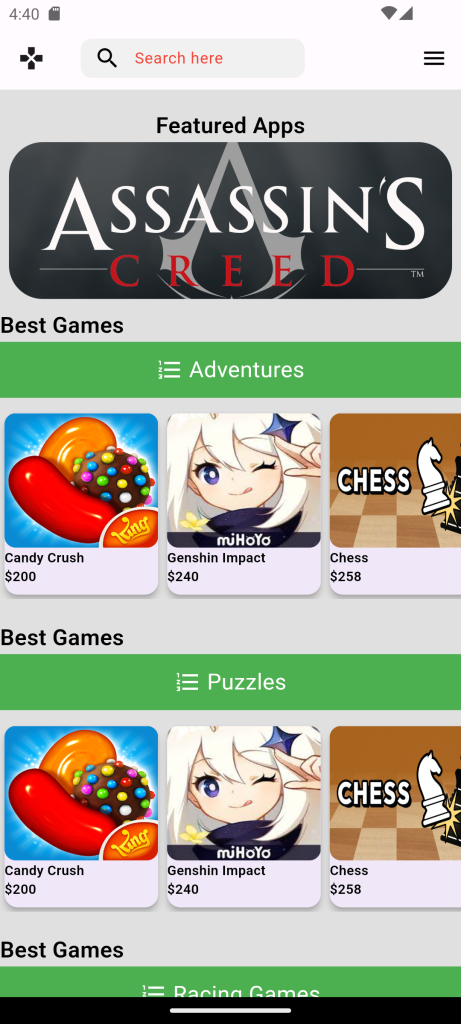
For More : To know about Delivery app Ui in Flutter.
Leave a Reply