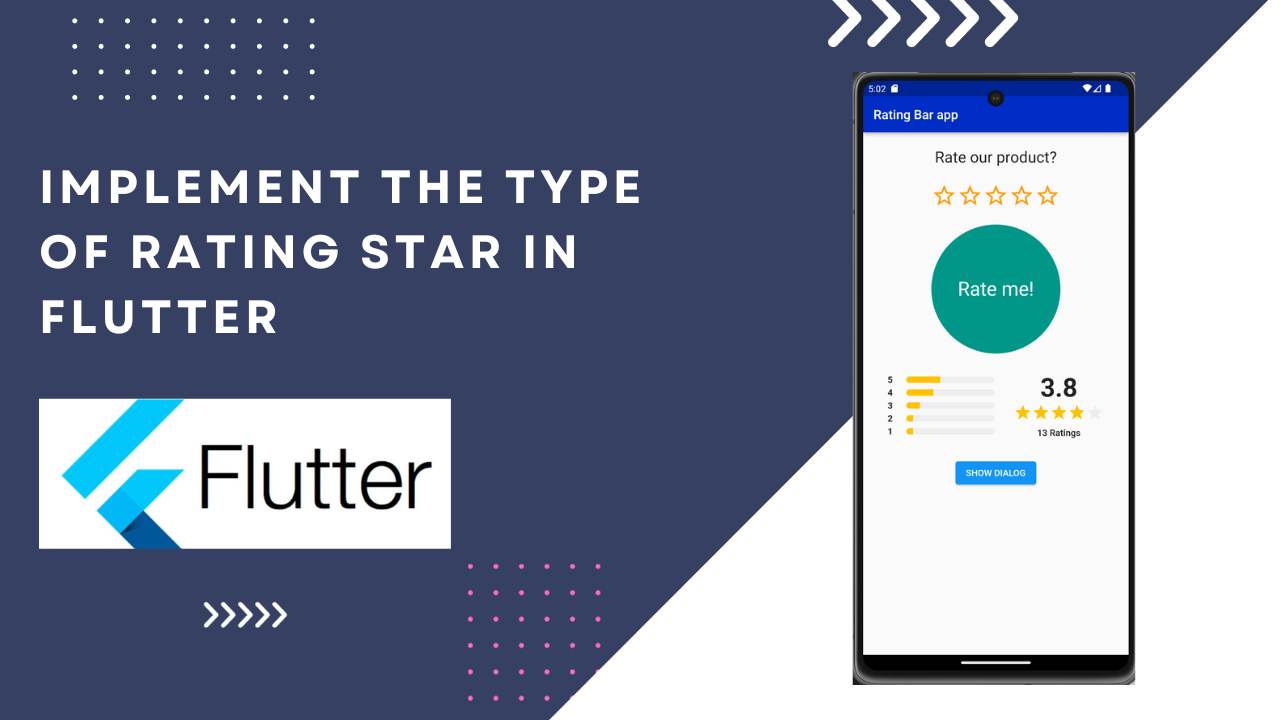
Implement Star Rating in Flutter:Most mobile applications and websites about e-commerce, ride-hailing, e-learning services, etc., have a function that allows users to rate products or services with the highest rating of 5 stars. or 10 stars.
In this article I will show you how to implement a rating bar in your Flutter application. A rating bar is generally composed of 5 stars and allow your user to rate a product, an article or anything else. You can also use a rating bar to show informations to your application user like fat, calories, prices of a receipe. What ever is your application subject you always need a rating bar somewhere in your Flutter application.
Install the dependency
First of all, create a small basic project to test rating bar dependency. I will show you step by step how to do this from a scratch project
Implement Star Rating in Flutter:Install the Plugin
1. Add flutter_rating_bar and its latest version to the dependencies section in your pubspec.yaml file by performing this:
flutter_rating_bar: ^4.0.1
rating_summary: ^1.0.2+1
2.Import it into your Dart code:
import 'package:flutter_rating_bar/flutter_rating_bar.dart';
Star rating bar code
RatingBar(
initialRating: 0,
direction: Axis.horizontal,
allowHalfRating: true,
itemCount: 5,
ratingWidget: RatingWidget(
full: const Icon(Icons.star, color: Colors.orange),
half: const Icon(
Icons.star_half,
color: Colors.orange,
),
empty: const Icon(
Icons.star_outline,
color: Colors.orange,
)),
onRatingUpdate: (value) {
setState(() {
_ratingValue = value;
});
}),
Rating summary code
RatingSummary(
counter: 13,
average: 3.846,
counterFiveStars: 5,
counterFourStars: 4,
counterThreeStars: 2,
counterTwoStars: 1,
counterOneStars: 1,
),
Dialog Rating code
The flutter_rating_bar package has a number of parameters that you can use to customize the appearance and behavior of the rating bar. Some of the most common parameters include:
- allowHalfRating: Whether or not to allow users to select half ratings.
- direction: The direction of the rating bar, either Axis.horizontal or Axis.vertical.
- itemCount: The number of rating items in the bar.
- itemSize: The size of each rating item.
- itemBuilder: A function that builds the rating items.
- minRating: The minimum rating that the user can select.
- maxRating: The maximum rating that the user can select.
- rating: The current rating of the bar.
- unratedColor: The color of the rating items when they are unrated.
showDialog(
context: context,
builder: (BuildContext context) => CupertinoAlertDialog(
title: const Padding(
padding: EdgeInsets.only(bottom: 8.0),
child: Text(
'Rating',
style: TextStyle(fontSize: 16),
),
),
content: Column(
children: [
RatingBar.builder(
initialRating: 5,
minRating: 1,
itemSize: 20,
direction: Axis.horizontal,
itemPadding: const EdgeInsets.symmetric(horizontal: 2.0),
itemBuilder: (context, _) => const Icon(
Icons.star,
color: Colors.amber,
),
onRatingUpdate: (rating) {},
),
],
),
actions: [
CupertinoDialogAction(
child: const Text(
"Cancel",
),
onPressed: () {
Navigator.of(context).pop();
},
),
CupertinoDialogAction(
child: const Text('Submit'),
onPressed: () {
Navigator.of(context).pop();
},
)
],
),
);
},
child: const Text("SHOW DIALOG"),
),
),
);
Full Code
import 'package:demo1/Constant/color.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter_rating_bar/flutter_rating_bar.dart';
import 'package:rating_summary/rating_summary.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Rating Bar app',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// The rating value
double? _ratingValue;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Blue,
title: const Text('Rating Bar app'),
),
body: Padding(
padding: const EdgeInsets.all(25),
child: Center(
child: Column(
children: [
const Text(
'Rate our product?',
style: TextStyle(fontSize: 24),
),
const SizedBox(height: 25),
// implement the rating bar
RatingBar(
initialRating: 0,
direction: Axis.horizontal,
allowHalfRating: true,
itemCount: 5,
ratingWidget: RatingWidget(
full: const Icon(Icons.star, color: Colors.orange),
half: const Icon(
Icons.star_half,
color: Colors.orange,
),
empty: const Icon(
Icons.star_outline,
color: Colors.orange,
)),
onRatingUpdate: (value) {
setState(() {
_ratingValue = value;
});
}),
const SizedBox(height: 25),
// Display the rate in number
Container(
width: 200,
height: 200,
decoration: const BoxDecoration(
color: Colors.teal, shape: BoxShape.circle),
alignment: Alignment.center,
child: Text(
_ratingValue != null ? _ratingValue.toString() : 'Rate me!',
style: const TextStyle(color: Colors.white, fontSize: 30),
),
),
SizedBox(
height: 30,
),
RatingSummary(
counter: 13,
average: 3.846,
counterFiveStars: 5,
counterFourStars: 4,
counterThreeStars: 2,
counterTwoStars: 1,
counterOneStars: 1,
),
SizedBox(
height: 30,
),
Center(
child: ElevatedButton(
onPressed: () {
showDialog(
context: context,
builder: (BuildContext context) => CupertinoAlertDialog(
title: const Padding(
padding: EdgeInsets.only(bottom: 8.0),
child: Text(
'Rating',
style: TextStyle(fontSize: 16),
),
),
content: Column(
children: [
RatingBar.builder(
initialRating: 5,
minRating: 1,
itemSize: 20,
direction: Axis.horizontal,
itemPadding:
const EdgeInsets.symmetric(horizontal: 2.0),
itemBuilder: (context, _) => const Icon(
Icons.star,
color: Colors.amber,
),
onRatingUpdate: (rating) {},
),
],
),
actions: [
CupertinoDialogAction(
child: const Text(
"Cancel",
),
onPressed: () {
Navigator.of(context).pop();
},
),
CupertinoDialogAction(
child: const Text('Submit'),
onPressed: () {
Navigator.of(context).pop();
},
)
],
),
);
},
child: const Text("SHOW DIALOG"),
),
),
],
),
),
));
}
}
For More Blog: Percent Indicator In Flutter
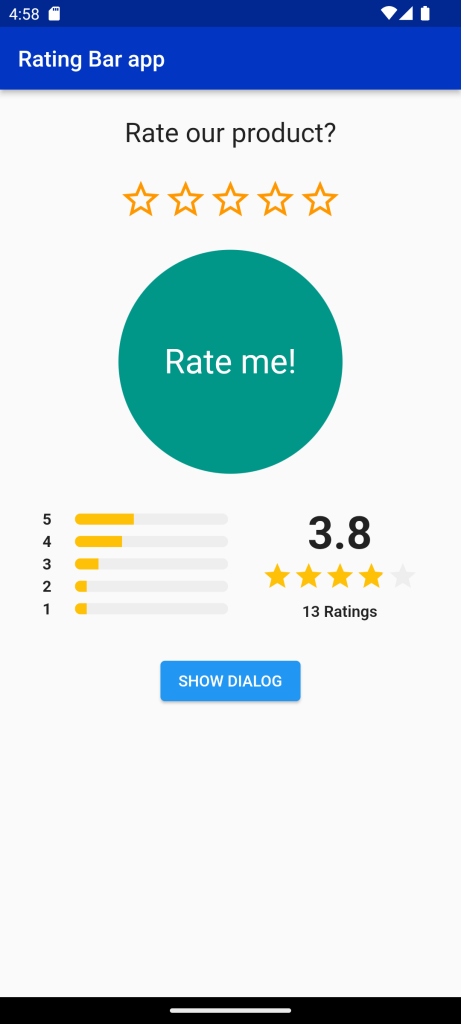
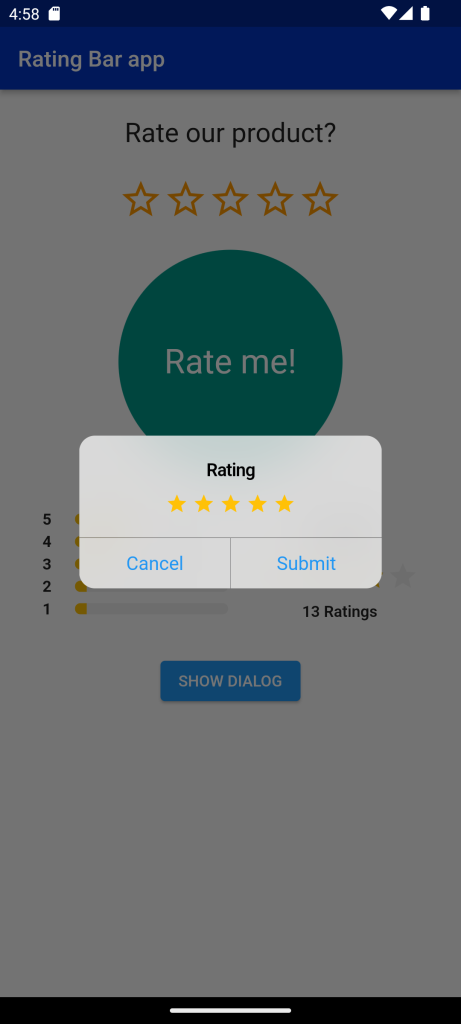
Leave a Reply